C#使用httpclient调用webservice接口
时间: 2023-09-18 20:14:38 浏览: 212
在 C# 中使用 `HttpClient` 调用 WebService 接口的方法如下:
1. 首先需要添加 `System.Net.Http` 和 `System.Web.Services` 引用。
2. 在代码中创建 `HttpClient` 实例,并设置请求头信息和请求内容。
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
using System.Xml;
namespace ConsoleApp1
{
class Program
{
static async Task Main(string[] args)
{
// 创建 HttpClient 实例
HttpClient httpClient = new HttpClient();
// 设置请求头信息
httpClient.DefaultRequestHeaders.Add("SOAPAction", "http://tempuri.org/HelloWorld");
// 设置请求内容
string soapRequest = @"<?xml version=""1.0"" encoding=""utf-8""?>
<soap:Envelope xmlns:soap=""http://schemas.xmlsoap.org/soap/envelope/"">
<soap:Body>
<HelloWorld xmlns=""http://tempuri.org/"" />
</soap:Body>
</soap:Envelope>";
HttpContent httpContent = new StringContent(soapRequest, System.Text.Encoding.UTF8, "text/xml");
// 发送请求
HttpResponseMessage httpResponse = await httpClient.PostAsync("http://localhost:8080/HelloWorldService.asmx", httpContent);
// 处理响应
if (httpResponse.IsSuccessStatusCode)
{
string soapResponse = await httpResponse.Content.ReadAsStringAsync();
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.LoadXml(soapResponse);
string result = xmlDoc.GetElementsByTagName("HelloWorldResult")[0].InnerText;
Console.WriteLine($"调用成功,返回结果:{result}");
}
else
{
Console.WriteLine($"调用失败,响应状态码:{httpResponse.StatusCode}");
}
}
}
}
```
上述代码以调用 HelloWorld 接口为例,可以根据实际情况更改请求头信息和请求内容。此外,我们还需要处理响应。如果响应状态码为成功,我们可以解析出响应内容,并获取接口返回的结果。
阅读全文
相关推荐
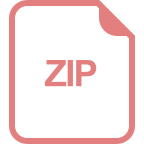

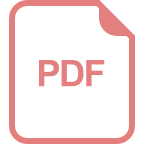
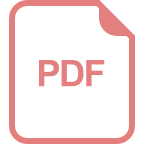
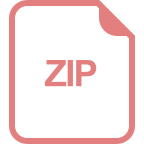
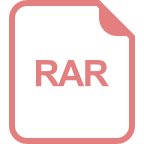
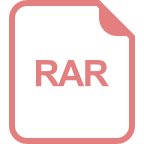
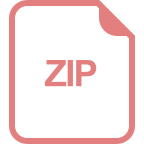
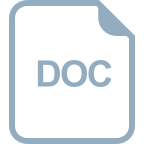



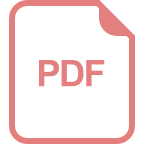
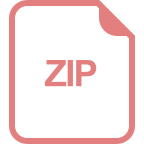