matlab 决策树怎样编写
时间: 2023-09-21 18:10:38 浏览: 79
在 MATLAB 中,可以使用 “Classification Learner App” 或 “fitctree” 函数来构建决策树。
1. 使用 Classification Learner App:
- 打开 MATLAB,并在命令窗口中输入 “ClassificationLearner”。
- 在弹出的窗口中选择要使用的数据集。
- 在左侧的 “Classification Model” 栏中选择 “Decision Trees”。
- 选择要使用的属性和标签。
- 点击 “Train” 按钮来训练模型。
- 可以使用 “Test” 按钮测试模型并进行预测。
2. 使用 fitctree 函数:
- 将属性和标签存储在矩阵中。
- 输入以下代码:
```matlab
tree = fitctree(X,Y);
view(tree,'Mode','graph');
```
其中,X 是属性矩阵,Y 是标签矩阵。
- 使用 view 函数可以可视化决策树。可以使用以下代码来显示决策树:
```matlab
view(tree,'Mode','graph');
```
这将显示一个决策树的可视化图形。
以上是两种常见的 MATLAB 决策树编写方法,你可以根据自己的需求选择其中一种。
相关问题
matlab决策树代码
以下是一个使用MATLAB编写的简单决策树代码示例:
```matlab
% 加载示例数据集(这里使用鸢尾花数据集)
load fisheriris
X = meas;
y = species;
% 将数据集拆分为训练集和测试集
rng(42); % 设置随机种子以确保结果可重现
cv = cvpartition(y, 'HoldOut', 0.2);
X_train = X(training(cv), :);
y_train = y(training(cv), :);
X_test = X(test(cv), :);
y_test = y(test(cv), :);
% 创建决策树模型
tree = fitctree(X_train, y_train);
% 在测试集上进行预测
y_pred = predict(tree, X_test);
% 计算准确率
accuracy = sum(strcmp(y_pred, y_test)) / numel(y_test);
disp(['准确率:', num2str(accuracy)]);
```
这段代码使用了MATLAB的`fitctree`函数来构建决策树模型,并使用鸢尾花数据集进行训练和测试。最后输出了模型在测试集上的准确率。
请注意,这只是一个简单的示例,实际应用中可能需要更多的预处理和调参步骤来优化模型性能。
决策树分类matlab
在 MATLAB 中,可以使用 Classification Learner App 或者编写代码来实现决策树分类。下面是一个使用代码实现的示例:
```matlab
% 加载数据集
load fisheriris
% 创建决策树模型
tree = fitctree(meas, species);
% 预测新样本
newData = [4.9, 3.1, 1.5, 0.1]; % 示例输入数据
predictedSpecies = predict(tree, newData);
disp(predictedSpecies);
```
在这个示例中,我们使用鸢尾花数据集(`fisheriris`)来训练一个决策树模型。然后,我们使用 `predict` 函数对新样本进行预测,并输出预测结果。
请注意,这只是一个简单的示例,实际应用中可能需要更多的数据预处理和调参工作。你可以根据自己的数据集和需求进行相应的修改和调整。
阅读全文
相关推荐
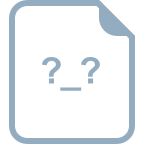
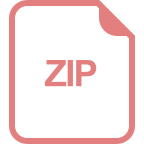
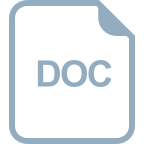
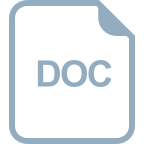
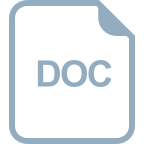
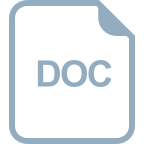
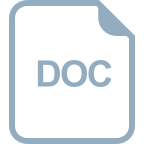
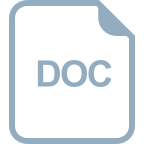
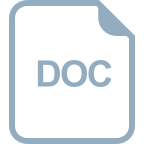
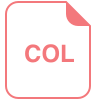



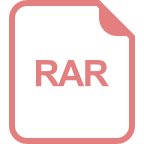