把腐蚀和膨胀算法函数用Python写出来
时间: 2024-05-15 20:12:46 浏览: 43
好的,以下是腐蚀和膨胀算法的Python实现:
腐蚀算法:
```python
import numpy as np
import cv2
def erosion(image, kernel):
# 获取图像和卷积核的大小
rows, cols = image.shape
k_rows, k_cols = kernel.shape
# 计算卷积核的中心位置
center_row = k_rows // 2
center_col = k_cols // 2
# 创建空白图像
eroded = np.zeros_like(image)
# 对每个像素进行操作
for i in range(rows):
for j in range(cols):
# 对于卷积核覆盖到的每个像素
for m in range(k_rows):
for n in range(k_cols):
# 计算卷积核中心点在图像上的位置
row = i + m - center_row
col = j + n - center_col
# 如果卷积核越界,则忽略该像素
if row < 0 or col < 0 or row >= rows or col >= cols:
continue
# 如果该像素与卷积核中心点重合,则判断是否需要腐蚀
if kernel[m, n] == 1 and image[row, col] == 0:
eroded[i, j] = 0
break
else:
continue
break
# 如果卷积核中的所有像素都与图像中的像素匹配,则进行腐蚀
else:
eroded[i, j] = 255
return eroded
```
膨胀算法:
```python
import numpy as np
import cv2
def dilation(image, kernel):
# 获取图像和卷积核的大小
rows, cols = image.shape
k_rows, k_cols = kernel.shape
# 计算卷积核的中心位置
center_row = k_rows // 2
center_col = k_cols // 2
# 创建空白图像
dilated = np.zeros_like(image)
# 对每个像素进行操作
for i in range(rows):
for j in range(cols):
# 对于卷积核覆盖到的每个像素
for m in range(k_rows):
for n in range(k_cols):
# 计算卷积核中心点在图像上的位置
row = i + m - center_row
col = j + n - center_col
# 如果卷积核越界,则忽略该像素
if row < 0 or col < 0 or row >= rows or col >= cols:
continue
# 如果该像素与卷积核中心点重合,则判断是否需要膨胀
if kernel[m, n] == 1 and image[row, col] == 255:
dilated[i, j] = 255
break
else:
continue
break
# 如果卷积核中的所有像素都与图像中的像素匹配,则进行膨胀
else:
dilated[i, j] = 0
return dilated
```
这里的 `image` 是输入图像, `kernel` 是卷积核。在这里,我们假设输入图像为二值图像,即只包含黑色和白色两种颜色。因此,腐蚀操作会将黑色部分腐蚀掉,而膨胀操作则会将白色部分膨胀。
阅读全文
相关推荐
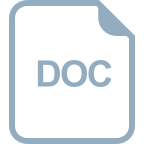
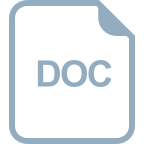
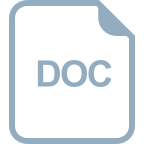

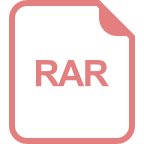
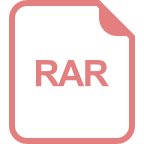
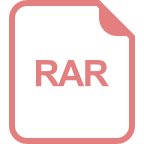
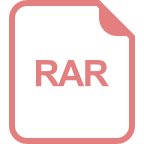
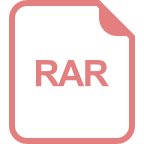
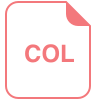


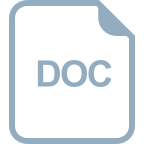
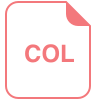
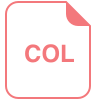
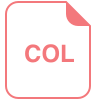
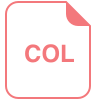
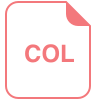
