vue中使用日历进行打卡签到是一个什么过程
时间: 2024-02-02 07:03:03 浏览: 25
在 Vue 中使用日历进行打卡签到的过程通常如下:
1. 安装日历组件库:在 Vue 中使用日历组件需要先安装相应的组件库,例如 FullCalendar、vue-fullcalendar、vue-simple-calendar 等等,可以通过 npm 或 yarn 安装。
2. 导入日历组件:在需要使用日历的 Vue 组件中导入相应的日历组件,并使用组件进行渲染。
3. 配置日历:在 Vue 组件中配置日历的参数,例如日历的初始日期、可选日期范围、事件等等。
4. 渲染打卡签到按钮:在日历中添加打卡签到按钮,并绑定相应的事件处理函数。
5. 处理打卡签到事件:在打卡签到按钮的事件处理函数中,根据当前日期和用户信息等数据,向后台发送签到请求,并根据返回结果更新页面状态。
6. 展示签到记录:在日历中展示用户的历史签到记录,可以通过自定义事件渲染函数或者插件等方式来实现。
总之,使用日历进行打卡签到的过程需要先安装相应的日历组件库,然后在 Vue 组件中进行配置和渲染,最后根据具体业务需求进行事件处理和数据展示。
相关问题
vue打卡日历_vue 日历签到
Vue可以通过使用第三方组件库或自己编写组件来实现日历签到功能。
1. 使用第三方组件库
例如,使用Element UI中的DatePicker组件,可以实现选择日期的功能。在选中日期后,可以将签到状态保存到后端数据库中,再在日历上展示已签到的日期。
2. 自己编写组件
可以使用Vue自带的组件和插件来编写日历签到组件。具体实现方法如下:
- 在Vue项目中创建一个Calendar组件;
- 使用Vue自带的v-for指令展示日历;
- 在每个日期上绑定点击事件,用来切换签到状态;
- 将签到状态保存到Vuex或后端数据库中;
- 在日历上展示已签到的日期。
下面是一个简单的日历签到组件代码示例:
```html
<template>
<div class="calendar">
<div class="header">{{ year }}年{{ month }}月</div>
<table>
<thead>
<tr>
<th v-for="day in days">{{ day }}</th>
</tr>
</thead>
<tbody>
<tr v-for="week in weeks">
<td v-for="date in week" :class="{ active: isSigned(date) }" @click="toggleSign(date)">{{ date }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
data() {
return {
year: new Date().getFullYear(),
month: new Date().getMonth() + 1,
days: ["日", "一", "二", "三", "四", "五", "六"],
weeks: [],
signedDates: []
};
},
computed: {
daysInMonth() {
const date = new Date(this.year, this.month, 0);
return date.getDate();
}
},
mounted() {
this.generateCalendar();
},
methods: {
generateCalendar() {
const firstDay = new Date(this.year, this.month - 1, 1).getDay();
const lastDate = this.daysInMonth;
const weeks = [];
let week = [];
for (let i = 0; i < firstDay; i++) {
week.push("");
}
for (let i = 1; i <= lastDate; i++) {
week.push(i);
if (week.length === 7) {
weeks.push(week);
week = [];
}
}
if (week.length > 0) {
for (let i = week.length; i < 7; i++) {
week.push("");
}
weeks.push(week);
}
this.weeks = weeks;
},
toggleSign(date) {
if (this.isSigned(date)) {
// 取消签到
const index = this.signedDates.indexOf(date);
this.signedDates.splice(index, 1);
} else {
// 签到
this.signedDates.push(date);
}
// 将签到状态保存到Vuex或后端数据库中
},
isSigned(date) {
return this.signedDates.includes(date);
}
}
};
</script>
<style>
.calendar {
width: 300px;
margin: 0 auto;
text-align: center;
}
.header {
font-size: 16px;
font-weight: bold;
margin-bottom: 10px;
}
table {
width: 100%;
border-collapse: collapse;
}
th {
height: 30px;
line-height: 30px;
}
td {
height: 50px;
line-height: 50px;
cursor: pointer;
}
td.active {
background-color: #66ccff;
color: #fff;
}
</style>
```
这个示例中的Calendar组件展示了一个月的日历,并提供了签到功能。你可以根据自己的需求进行修改和扩展。
vue3打卡日历_vue3 日历签到
Vue3 打卡日历是一个基于 Vue3 开发的日历组件,可以方便地展示月视图和周视图,并支持日历签到功能。
首先,在你的 Vue3 项目中安装 `vue3-calendar`:
```
npm i vue3-calendar
```
然后在需要使用日历组件的地方引入:
```html
<template>
<div>
<Calendar :events="events" :signed="signed" @event-click="onEventClick" @date-click="onDateClick" />
</div>
</template>
<script>
import Calendar from 'vue3-calendar'
export default {
components: {
Calendar
},
data () {
return {
events: [
{ title: 'Event 1', date: '2022-01-01' },
{ title: 'Event 2', date: '2022-01-15' },
{ title: 'Event 3', date: '2022-01-20' }
],
signed: ['2022-01-01', '2022-01-15']
}
},
methods: {
onEventClick (event) {
console.log(event)
},
onDateClick (date) {
console.log(date)
}
}
}
</script>
```
在上面的代码中,我们将 `vue3-calendar` 引入并注册为 `Calendar` 组件。然后在模板中使用 `<Calendar>` 标签,并传入两个属性:
- `events`:用于指定日历上显示的事件列表,每个事件包含 `title` 和 `date` 两个属性;
- `signed`:用于指定哪些日期已经签到。
`<Calendar>` 组件还提供了两个事件:
- `event-click`:当用户点击某个事件时触发,可以通过 `$event` 参数获取被点击的事件信息;
- `date-click`:当用户点击某个日期时触发,可以通过 `$event` 参数获取被点击的日期信息。
最后,你需要在样式中引入日历组件的 CSS 文件:
```html
<style>
@import 'vue3-calendar/dist/vue3-calendar.css';
</style>
```
相关推荐
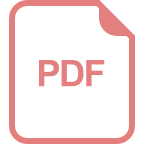












