html +css 实现一个自动循环滚动的表格,鼠标悬浮时停止,并且包含详情点击按钮
时间: 2023-12-03 15:42:19 浏览: 160
我们可以使用 JavaScript 和 CSS 实现一个自动循环滚动的表格,当鼠标悬停时停止滚动,并在每行的最后一列添加一个详情按钮。
HTML 结构:
```html
<div class="table-container">
<table>
<thead>
<tr>
<th>Column 1</th>
<th>Column 2</th>
<th>Column 3</th>
<th>Details</th>
</tr>
</thead>
<tbody id="table-body">
<tr>
<td>Row 1, Column 1</td>
<td>Row 1, Column 2</td>
<td>Row 1, Column 3</td>
<td><button class="details-btn">Details</button></td>
</tr>
<tr>
<td>Row 2, Column 1</td>
<td>Row 2, Column 2</td>
<td>Row 2, Column 3</td>
<td><button class="details-btn">Details</button></td>
</tr>
<tr>
<td>Row 3, Column 1</td>
<td>Row 3, Column 2</td>
<td>Row 3, Column 3</td>
<td><button class="details-btn">Details</button></td>
</tr>
<!-- Add more rows here -->
</tbody>
</table>
</div>
```
CSS 样式:
```css
.table-container {
width: 100%;
overflow: hidden;
}
table {
width: 100%;
border-collapse: collapse;
table-layout: fixed;
}
thead th,
tbody td {
padding: 8px;
text-align: left;
border: 1px solid #ddd;
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
}
.details-btn {
background-color: #4CAF50;
color: white;
border: none;
padding: 8px 16px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 14px;
cursor: pointer;
}
.details-btn:hover {
background-color: #3e8e41;
}
```
JavaScript 代码:
```js
const tableBody = document.getElementById("table-body");
const rows = tableBody.getElementsByTagName("tr");
let currentRow = 0;
let intervalId = null;
function startScroll() {
intervalId = setInterval(() => {
rows[currentRow].classList.remove("active");
currentRow++;
if (currentRow >= rows.length) {
currentRow = 0;
}
rows[currentRow].classList.add("active");
}, 2000);
}
function stopScroll() {
clearInterval(intervalId);
}
for (const row of rows) {
const detailsBtn = row.querySelector(".details-btn");
detailsBtn.addEventListener("click", () => {
// Handle the details button click
console.log("Details clicked for row:", row);
});
}
tableBody.addEventListener("mouseover", stopScroll);
tableBody.addEventListener("mouseout", startScroll);
startScroll();
```
我们首先获取表格的 tbody 元素和其中的所有行,然后定义一个变量 currentRow 来追踪当前显示的行。我们使用 setInterval 函数在每 2 秒钟更新 currentRow 的值,并将当前行添加一个 active 类来突出显示它。如果我们到达最后一行,我们将 currentRow 重置为 0。
我们还定义了两个函数,startScroll 和 stopScroll,用于启动和停止滚动。我们使用 setInterval 函数来自动滚动表格,但是当鼠标悬停在表格上时,我们需要停止滚动。我们通过添加 mouseover 和 mouseout 事件监听器来实现这一点。
最后,我们为每个详情按钮添加一个点击事件监听器,并在点击时处理该行的详细信息。
阅读全文
相关推荐
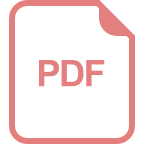
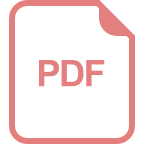
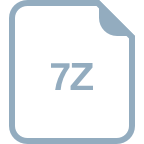













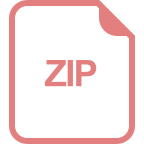
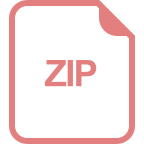