怎么让BOOL数组所有值为true
时间: 2023-11-11 08:06:46 浏览: 344
在 C# 中,可以使用 `Enumerable.Repeat` 方法来创建指定大小且所有元素都为 True 的 bool 数组。例如,如果要创建一个大小为 5 且所有元素都为 True 的 bool 数组,可以使用以下代码:
```
bool[] arr = Enumerable.Repeat(true, 5).ToArray();
```
在上面的代码中,我们使用 `Enumerable.Repeat` 方法创建一个大小为 5 的 bool 数组,并将所有元素都设置为 True,然后使用 `ToArray` 方法将其转换为数组。
你也可以使用 `for` 循环将数组的每个元素设置为 True。例如,如果有一个名为 `boolArray` 的 bool 数组,可以使用以下代码将其所有元素都设置为 True:
```
for (int i = 0; i < boolArray.Length; i++)
{
boolArray[i] = true;
}
```
在上面的代码中,我们使用 `for` 循环遍历数组的每个元素,并将其设置为 True。
相关问题
我有一个bool数组,怎么得到它所有为true的索引加一的值?
你可以使用LINQ(Language Integrated Query)中的`Select`和`Where`方法来达到这个目的。以下是一个简单的示例:
```csharp
using System;
using System.Linq;
public class Program
{
public static void Main()
{
bool[] array = new bool[] { true, false, true, false, true };
int[] indexesPlusOne = array.Select((value, index) => value ? index + 1 : default(int))
.Where(index => index != default(int)) // 过滤掉null值(false对应的)
.ToArray();
Console.WriteLine("True indices plus one:");
foreach (int index in indexesPlusOne)
{
Console.Write(index + " ");
}
}
}
```
这段代码首先创建了一个布尔数组,然后使用`Select`方法,当元素为真时返回索引+1,否则返回默认值`default(int)`(因为非真的值不会映射到索引)。接着用`Where`过滤掉默认值,最后将结果存储在一个新的整数数组中并打印出来。
怎么判断bool数组中True的个数
可以使用Python中的count()函数来统计bool数组中True的个数,示例代码如下:
```python
bool_list = [True, False, True, True, False]
true_count = bool_list.count(True)
print(true_count) # 输出结果为:3
```
或者也可以使用循环遍历数组,逐个判断元素是否为True,并计数,示例代码如下:
```python
bool_list = [True, False, True, True, False]
true_count = 0
for element in bool_list:
if element:
true_count += 1
print(true_count) # 输出结果为:3
```
两种方法都可以得到bool数组中True的个数。
阅读全文
相关推荐
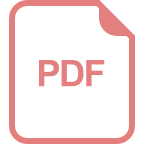
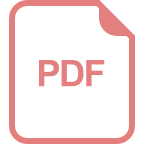
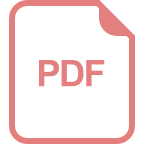













