智能指针的实现
时间: 2023-07-20 14:06:06 浏览: 56
智能指针是一种 C++ 的语言特性,它可以自动管理动态分配的内存。实现一个智能指针需要考虑以下几个方面:
1. 引用计数:智能指针需要记录当前有多少个指针指向同一个对象,以便于在没有指针指向这个对象时能够正确地释放内存。
2. 拷贝和赋值:智能指针的拷贝和赋值需要正确地更新引用计数,以避免出现两个指针同时指向同一个对象但引用计数不正确的情况。
3. 析构函数:当智能指针的引用计数为零时需要正确地释放内存,以避免内存泄漏。
下面是一个简单的智能指针实现:
```cpp
template <typename T>
class SmartPointer {
public:
SmartPointer() : m_ptr(nullptr), m_ref_count(new int(0)) {}
SmartPointer(T *ptr) : m_ptr(ptr), m_ref_count(new int(1)) {}
SmartPointer(const SmartPointer<T> &other) : m_ptr(other.m_ptr), m_ref_count(other.m_ref_count) {
(*m_ref_count)++;
}
~SmartPointer() {
(*m_ref_count)--;
if (*m_ref_count == 0) {
delete m_ptr;
delete m_ref_count;
}
}
SmartPointer<T> &operator=(const SmartPointer<T> &other) {
if (this != &other) {
(*m_ref_count)--;
if (*m_ref_count == 0) {
delete m_ptr;
delete m_ref_count;
}
m_ptr = other.m_ptr;
m_ref_count = other.m_ref_count;
(*m_ref_count)++;
}
return *this;
}
T &operator*() const {
return *m_ptr;
}
T *operator->() const {
return m_ptr;
}
private:
T *m_ptr;
int *m_ref_count;
};
```
这个实现使用一个指向 int 的指针来记录引用计数。在构造函数中,如果传入了一个指针,则将引用计数初始化为 1,否则将其初始化为 0。在拷贝构造函数中,需要正确地更新引用计数。在析构函数中,如果引用计数为零则释放内存。在赋值操作符中,需要正确地更新引用计数。
相关推荐
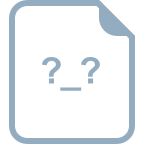
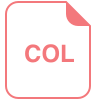
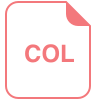
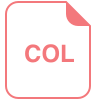
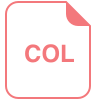
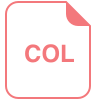









