c++如何用智能指针实现链表的几种方式,包括用unique_ptr实现、shared_ptr实现
时间: 2023-06-27 11:06:06 浏览: 65
链表是一种常见的数据结构,智能指针可以帮助我们管理链表节点的内存,避免内存泄漏和野指针的问题。下面介绍几种用智能指针实现链表的方式。
1. 用unique_ptr实现
unique_ptr是C++11标准引入的智能指针,每个unique_ptr对象都“独占”所指向的内存资源,即同一时间只能有一个unique_ptr对象拥有该内存资源,其他unique_ptr对象无法访问该内存资源。因此,我们可以用unique_ptr实现链表节点,每个节点拥有唯一的unique_ptr对象。
```c++
#include <memory>
template<typename T>
struct Node {
T data;
std::unique_ptr<Node<T>> next;
Node(T data) : data(std::move(data)), next(nullptr) {}
};
template<typename T>
class LinkedList {
public:
LinkedList() : head(nullptr) {}
void insert(T data) {
std::unique_ptr<Node<T>> newNode = std::make_unique<Node<T>>(std::move(data));
if (!head) {
head = std::move(newNode);
} else {
Node<T>* current = head.get();
while (current->next) {
current = current->next.get();
}
current->next = std::move(newNode);
}
}
private:
std::unique_ptr<Node<T>> head;
};
```
2. 用shared_ptr实现
shared_ptr是C++11标准引入的智能指针,多个shared_ptr对象可以“共享”同一个内存资源,即同一时间可以有多个shared_ptr对象拥有该内存资源。因此,我们可以用shared_ptr实现链表节点,每个节点拥有一个shared_ptr对象,多个节点的shared_ptr对象可以共享同一个内存资源。
```c++
#include <memory>
template<typename T>
struct Node {
T data;
std::shared_ptr<Node<T>> next;
Node(T data) : data(std::move(data)), next(nullptr) {}
};
template<typename T>
class LinkedList {
public:
LinkedList() : head(nullptr) {}
void insert(T data) {
std::shared_ptr<Node<T>> newNode = std::make_shared<Node<T>>(std::move(data));
if (!head) {
head = std::move(newNode);
} else {
std::shared_ptr<Node<T>> current = head;
while (current->next) {
current = current->next;
}
current->next = std::move(newNode);
}
}
private:
std::shared_ptr<Node<T>> head;
};
```
以上是用智能指针实现链表的两种方式,其中unique_ptr和shared_ptr都可以有效避免内存泄漏和野指针的问题。选择哪种方式取决于具体需求和场景,需要根据实际情况进行选择。
相关推荐
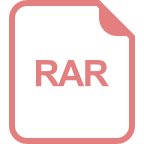
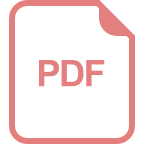














