用C语言数组方法存储并显示学生的学号、姓名,班级,高等数学成绩、英语成绩、数据结构成绩、平均分和总分等内容。
时间: 2024-12-06 08:26:24 浏览: 56
在C语言中,你可以使用一维数组来存储学生的信息,并通过循环结构来遍历和显示这些信息。首先,定义一个结构体`Student`来包含学生的各项属性:
```c
typedef struct {
int student_id; // 学号
char name[50]; // 姓名
char class_name[50]; // 班级
float math_score; // 高等数学成绩
float english_score; // 英语成绩
float data_structure_score; // 数据结构成绩
} Student;
```
然后,创建一个数组来存放多个学生的信息:
```c
const int NUM_OF_STUDENTS = 5; // 假设有5个学生
Student students[NUM_OF_STUDENTS];
```
接下来,可以填充数据并显示:
```c
void input_student_info(Student students[]) {
for (int i = 0; i < NUM_OF_STUDENTS; i++) {
printf("请输入第%d个学生的学号、姓名、班级以及各科成绩:\n", i + 1);
scanf("%d %s %s", &students[i].student_id, students[i].name, students[i].class_name);
scanf("%f %f %f", &students[i].math_score, &students[i].english_score, &students[i].data_structure_score);
}
}
void display_student_info(Student students[], int num_of_students) {
printf("学生信息:\n");
for (int i = 0; i < num_of_students; i++) {
printf("学号:%d,姓名:%s,班级:%s\n", students[i].student_id, students[i].name, students[i].class_name);
printf("高等数学:%.2f,英语:%.2f,数据结构:%.2f\n", students[i].math_score, students[i].english_score, students[i].data_structure_score);
// 计算平均分和总分
float total_scores = students[i].math_score + students[i].english_score + students[i].data_structure_score;
float average = total_scores / 3;
printf("平均分:%.2f,总分:%.2f\n", average, total_scores);
printf("\n");
}
}
```
最后,结合这两个函数,可以在主程序中调用它们:
```c
int main() {
input_student_info(students);
display_student_info(students, NUM_OF_STUDENTS);
return 0;
}
```
阅读全文
相关推荐
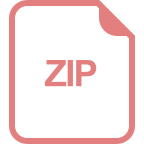
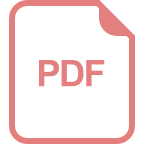
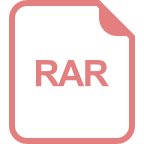



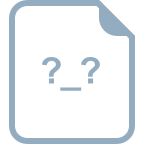
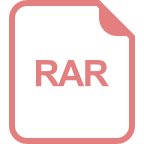
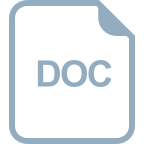
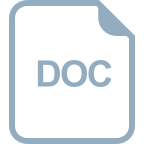
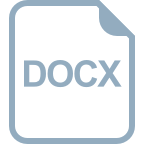
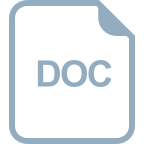
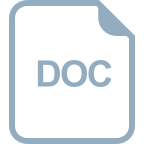
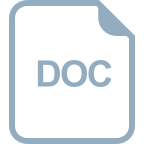
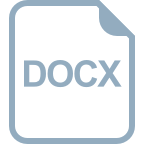
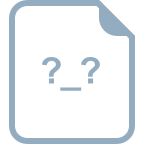