用python设计一个仿真实验室内仪器之间传动关系的系统
时间: 2024-11-13 22:39:33 浏览: 12
在Python中设计一个仿真实验室内仪器之间的传动关系系统,通常会利用面向对象编程(OOP)的概念以及一些库如Pygame或matplotlib来模拟物理交互。以下是一个简化的例子:
```python
import pygame
from pygame.locals import *
# 设定基本参数
class Instrument:
def __init__(self, name, position):
self.name = name
self.position = position
self.speed = 0
# 模拟传动函数,这里简化为速度传递
def transmit(self, other_instrument):
if hasattr(other_instrument, 'apply_speed'):
other_instrument.apply_speed(self.speed)
class Motor(Instrument):
def apply_speed(self, speed):
print(f"{self.name}向{other_instrument.name}传输了速度 {speed}")
class Lever(Instrument):
pass # Levers don't transmit directly but can be connected to motors
# 创建实例
motor_1 = Motor("电机1", (50, 50))
lever_1 = Lever("杠杆1", (100, 50))
motor_2 = Motor("电机2", (50, 100))
# 连接仪器
lever_1.transmit(motor_2)
# 渲染游戏循环
pygame.init()
screen = pygame.display.set_mode((400, 400))
clock = pygame.time.Clock()
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
# 更新并绘制每个仪器的位置
motor_1.position = (motor_1.position[0] + motor_1.speed, motor_1.position[1])
lever_1.position = (lever_1.position[0] + motor_1.speed, lever_1.position[1])
screen.fill((255, 255, 255))
pygame.draw.circle(screen, (0, 0, 0), motor_1.position, 10)
pygame.draw.circle(screen, (0, 0, 0), lever_1.position, 10)
pygame.display.flip()
clock.tick(60) # 控制帧率
```
这个例子创建了一个简单的系统,其中电机通过杠杆传递动力。在实际应用中,你可以添加更复杂的传动模型、摩擦力等物理特性。
阅读全文
相关推荐
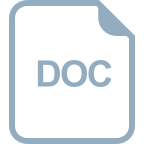
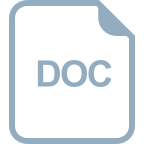
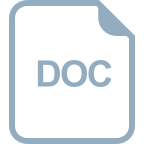
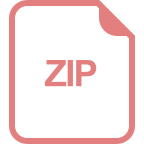
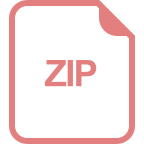
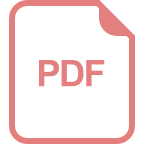
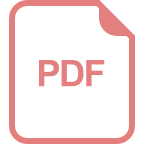
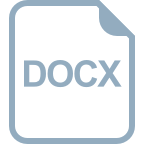
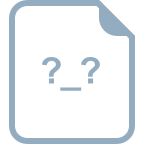
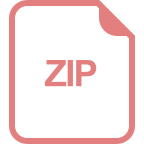
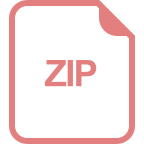
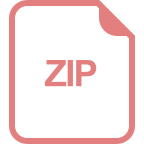
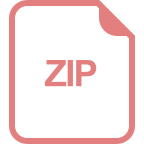
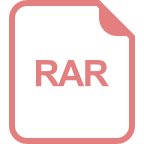
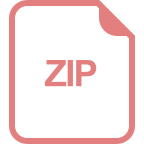
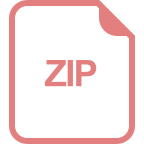
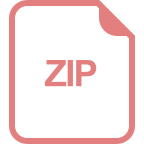
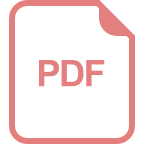
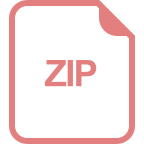