广度优先八皇后python
时间: 2023-10-19 20:31:58 浏览: 101
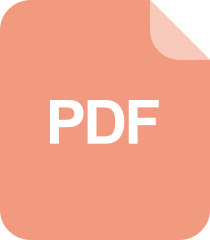
python 递归深度优先搜索与广度优先搜索算法模拟实现
要实现广度优先搜索算法解决八皇后问题,可以使用队列来存储待处理的状态。下面是一种可能的实现方法:
1. 定义一个Node类,用于表示每个状态。该类包含三个属性:board表示当前棋盘的状态,queens表示已放置的皇后数量,以及path表示从初始状态到当前状态的路径。
2. 创建一个空队列,并将初始状态(一个空棋盘)加入队列。
3. 进入循环,直到队列为空:
a. 从队列中取出一个状态,记为current。
b. 如果current中的queens等于8,表示已经找到了一个解法,输出current的path即可。
c. 否则,对current进行扩展,生成所有可能的下一步状态,并将这些状态加入队列。扩展的过程可以通过在current的棋盘上尝试放置一个皇后来实现。
d. 重复步骤a。
4. 如果循环结束时仍未找到解法,则说明无解。
以下是一个示例实现的Python代码:
```python
from collections import deque
class Node:
def __init__(self, board, queens, path):
self.board = board
self.queens = queens
self.path = path
def breadth_first_search():
initial_state = Node([None]*8, 0, [])
queue = deque()
queue.append(initial_state)
while queue:
current = queue.popleft()
if current.queens == 8:
return current.path
for i in range(8):
if is_valid(current.board, i, current.queens):
new_board = current.board.copy()
new_board[current.queens = i
new_queens = current.queens + 1
new_path = current.path + [i]
new_state = Node(new_board, new_queens, new_path)
queue.append(new_state)
return None
def is_valid(board, col, row):
for i in range(row):
if board[i == col or abs(board[i - col) == row - i:
return False
return True
solution = breadth_first_search()
if solution:
print(solution)
else:
print("No solution found.")
```
这段代码使用了一个Node类来表示状态,并通过广度优先搜索算法进行遍历。在search函数中,使用队列实现了广度优先搜索的过程。在每一步中,生成下一步的状态并将其加入队列。如果最终找到一个解法,就返回该解法的路径;否则,返回None表示无解。
<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [Python(广度优先搜索+回溯+递归)、lingo实现八皇后问题](https://blog.csdn.net/m0_62410163/article/details/130537889)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *3* [python实现八皇后问题与遇见的问题(使用深度优先bfs和广度优先dfs)](https://blog.csdn.net/qq_43471945/article/details/127855564)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
阅读全文
相关推荐
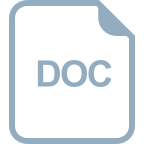
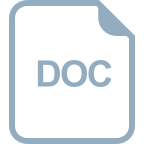
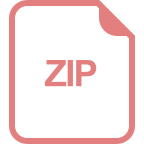
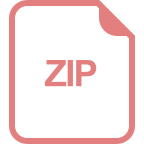
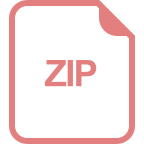
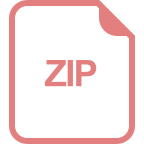
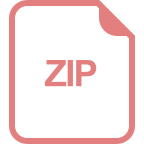
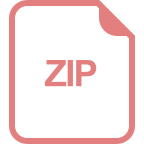
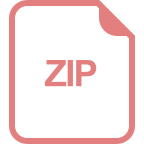
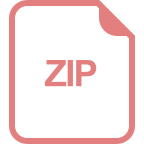
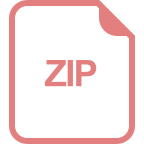
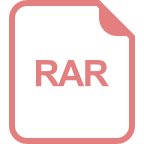
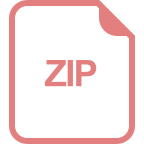
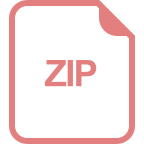
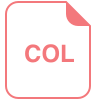
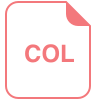

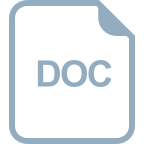