pyqt5设计计算器那个+-符号逻辑怎么写
时间: 2024-09-25 07:08:25 浏览: 27
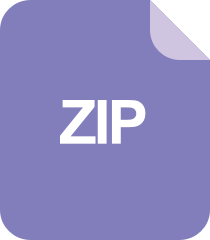
基于python+PYQT5的简易计算器源码+GUI界面.zip
在PyQt5中设计一个计算器应用,处理"+"和"-"这样的运算符通常涉及到事件绑定和简单的算术操作。当你用户点击加号或减号时,你需要捕获这个事件,然后触发相应的计算操作。以下是一个简化的示例:
```python
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QLineEdit, QVBoxLayout
from PyQt5.QtCore import Qt
class Calculator(QWidget):
def __init__(self):
super().__init__()
self.init_ui()
def init_ui(self):
layout = QVBoxLayout()
# 创建两个输入框,一个用于显示数字,一个用于存储操作数
self.display = QLineEdit("0", self)
self.display.setReadOnly(True)
self.current_number = QLineEdit("0", self) # 存储当前的数字
self.current_number.returnPressed.connect(self.handle_number_input)
buttons_layout = [
["7", "8", "9", "+"],
["4", "5", "6", "-"],
["1", "2", "3", "*"],
["0", ".", "=", "/"]
]
for row in buttons_layout:
button_row = QHBoxLayout()
for button_text in row:
button = QPushButton(button_text, self)
if button_text.isdigit():
button.clicked.connect(lambda x, txt=button_text: self.update_display(txt))
elif button_text == "=":
button.clicked.connect(self.calculate)
else:
button.clicked.connect(self.handle_operator)
button_row.addWidget(button)
layout.addLayout(button_row)
layout.addWidget(self.current_number)
self.setLayout(layout)
def handle_number_input(self):
text = self.current_number.text()
if text != "":
self.display.setText(text + ".")
self.current_number.clear()
def update_display(self, number):
self.current_number.setText(self.current_number.text() + number)
def handle_operator(self):
operator = self.current_number.text()
first_num = float(self.display.text())
self.current_number.clear()
self.display.setText(str(first_num))
if operator in ['+', '-']:
second_num = float(self.display.text())
result = self.apply_operation(first_num, second_num, operator)
self.display.setText(str(result))
def apply_operation(self, num1, num2, op):
if op == '+':
return num1 + num2
elif op == '-':
return num1 - num2
def calculate(self):
try:
result = eval(self.display.text())
self.display.setText(f"{result:.2f}")
except Exception as e:
self.display.setText("Error")
if __name__ == "__main__":
app = QApplication([])
calc = Calculator()
calc.show()
app.exec_()
```
在这个例子中,`handle_operator`方法负责处理"+"和"-"按钮点击,它会获取当前显示的数字、保存为操作数,然后清空显示框,接着进行相应运算并将结果显示回去。注意这里使用了Python内置的`eval`函数来直接解析表达式,但在实际项目中,更好的做法是使用`eval`的替代方案,因为`eval`存在安全风险。
阅读全文
相关推荐
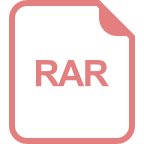
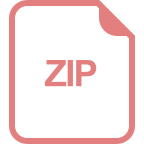
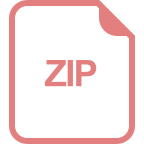
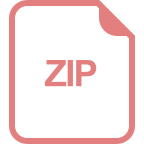
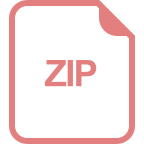
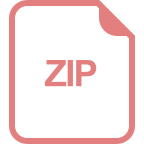
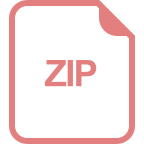
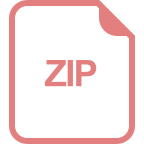
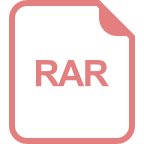
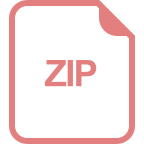
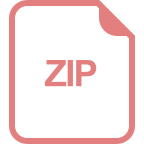
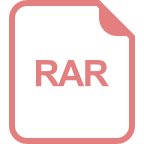
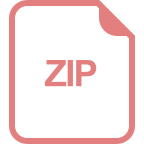
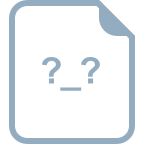