使用urllib3爬取人民邮电出版社图书检索页。检索关键字为网络爬虫
时间: 2024-10-10 22:11:19 浏览: 38
使用`urllib3`库来爬取人民邮电出版社的图书检索页面,首先需要安装这个库(如果你还没有安装的话,可以使用`pip install urllib3[secure]`),然后按照以下步骤操作:
1. 导入所需的库:
```python
import requests
from urllib3.util.retry import Retry
from requests.adapters import HTTPAdapter
```
2. 设置请求头,模拟浏览器访问以避免被识别为爬虫:
```python
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
```
3. 构造基础URL,添加搜索参数(这里假设书籍检索的API接口格式):
```python
base_url = "http://search.phei.com.cn/api/search"
params = {
'keyword': '网络爬虫',
'type': 'book', # 搜索类型,这里指书籍
'page': 1 # 开始爬取第一页数据
}
```
4. 使用`requests`配合`urllib3`的重试机制处理网络异常:
```python
retries = Retry(total=3, backoff_factor=0.5, status_forcelist=[500, 502, 503, 504])
adapter = HTTPAdapter(max_retries=retries)
session = requests.Session()
session.mount('http://', adapter)
session.mount('https://', adapter)
response = session.get(base_url, params=params, headers=headers)
```
5. 验证响应状态并处理获取的数据,通常会有一个JSON格式的返回结果,包含图书信息:
```python
if response.status_code == 200:
data = response.json()
books = data['result']['items']
for book in books:
print(book['title'], book['author'])
else:
print(f"Failed to fetch data, status code: {response.status_code}")
```
阅读全文
相关推荐
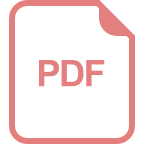
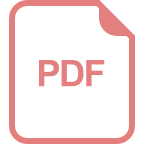
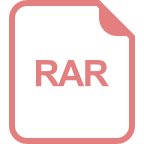

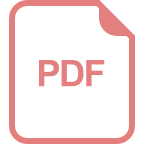
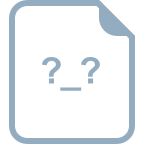
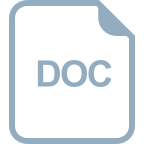












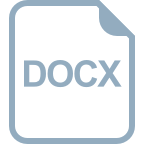