在c语言中,从键盘输入三个整数,分别代表年月日,输出这一天是该年的第几天
时间: 2024-10-19 13:16:06 浏览: 71
在C语言中,你可以通过以下步骤实现这个功能:
首先,你需要编写一个程序接收用户的输入,然后使用闰年规则来计算给定日期是一年中的第几天。闰年的判断条件通常是能被4整除但不能被100整除,除非它同时也能被400整除。
这里是一个简单的示例:
```c
#include <stdio.h>
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int days_in_month(int month, int year) {
switch(month) {
case 1: case 3: case 5: case 7: case 8: case 10: case 12:
return 31;
case 4: case 6: case 9: case 11:
return 30;
default: // February
if(is_leap_year(year)) {
return 29;
} else {
return 28;
}
}
}
int main() {
int year, month, day;
printf("请输入年份、月份和日期:");
scanf("%d %d %d", &year, &month, &day);
// 确保输入的是有效的日期
if(month <= 0 || month > 12 || day <= 0 || day > days_in_month(month, year)) {
printf("无效的日期。\n");
return 1;
}
int total_days = 0;
for (int i = 1; i < month; i++) {
total_days += days_in_month(i, year);
}
total_days += day;
printf("这一天是%ld年中的第%d天.\n", year, total_days);
return 0;
}
```
当你运行这个程序并输入年月日时,它会计算出这一天是一年中的第几天,并打印结果。
阅读全文
相关推荐
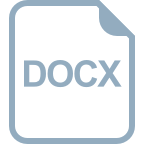
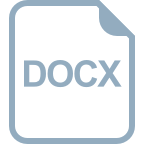
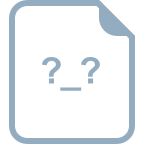
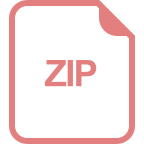
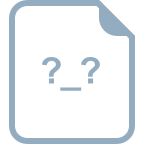
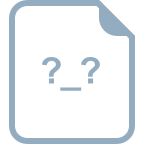
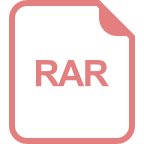
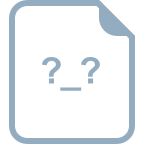
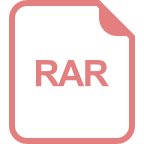
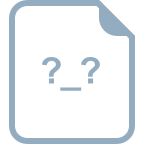
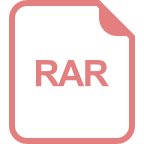
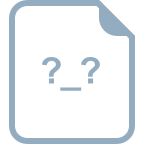
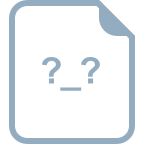
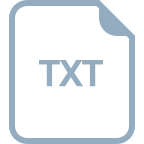
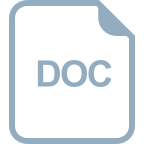