unity快速排序非递归
时间: 2023-09-27 14:08:43 浏览: 89
在Unity中,你可以使用非递归方法来实现快速排序算法。下面是一个示例代码:
```c#
using System.Collections.Generic;
public class QuickSort
{
public static void Sort(List<int> array)
{
if (array.Count <= 1)
return;
int left = 0;
int right = array.Count - 1;
Stack<int> stack = new Stack<int>();
stack.Push(left);
stack.Push(right);
while (stack.Count > 0)
{
right = stack.Pop();
left = stack.Pop();
int pivotIndex = Partition(array, left, right);
if (pivotIndex - 1 > left)
{
stack.Push(left);
stack.Push(pivotIndex - 1);
}
if (pivotIndex + 1 < right)
{
stack.Push(pivotIndex + 1);
stack.Push(right);
}
}
}
private static int Partition(List<int> array, int left, int right)
{
int pivot = array[right];
int i = left - 1;
for (int j = left; j < right; j++)
{
if (array[j] <= pivot)
{
i++;
Swap(array, i, j);
}
}
Swap(array, i + 1, right);
return i + 1;
}
private static void Swap(List<int> array, int i, int j)
{
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
```
你可以调用 `QuickSort.Sort()` 方法来对整数列表进行快速排序。请注意,这里使用了一个栈来模拟递归调用,避免使用递归的方法。
阅读全文
相关推荐
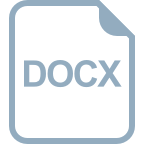
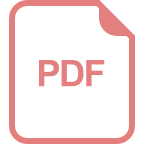
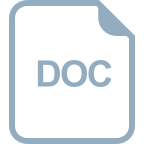
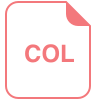
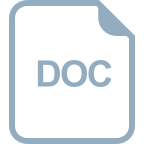
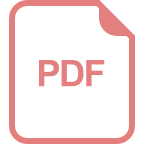
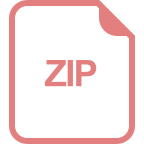
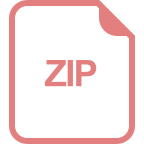
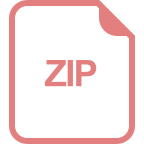
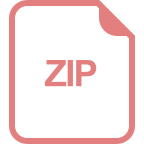
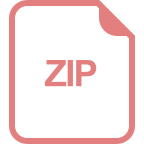
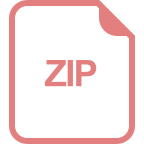