for i in range(self.stage_num): self.layers.append(BasicLayer(dim=embed_dims[i], depth=depths[i], net_depth=net_depth, kernel_size=kernel_size, conv_layer=conv_layer, norm_layer=norm_layer, gate_act=gate_act))解释每一段代码
时间: 2023-03-25 12:01:18 浏览: 67
这段代码是一个循环语句,其中self.stage_num是循环的次数。在每次循环中,会调用BasicLayer类,并将其实例化为一个对象,该对象的各个属性值由参数指定。具体来说,dim表示嵌入维度,depth表示层数,net_depth表示网络深度,kernel_size表示卷积核大小,conv_layer表示卷积层类型,norm_layer表示归一化层类型,gate_act表示门控激活函数类型。每次循环结束后,将该对象添加到layers列表中。
相关问题
def _freeze_stages(self): if self.frozen_stages >= 0: self.patch_embed.eval() for param in self.patch_embed.parameters(): param.requires_grad = False if self.frozen_stages >= 1: self.pos_drop.eval() for i in range(0, self.frozen_stages): m = self.layers[i] m.eval() for param in m.parameters(): param.requires_grad = False
这段代码是一个私有方法 `_freeze_stages`,用于冻结模型的前几层,以便进行迁移学习或微调。具体来说,如果 `frozen_stages` 大于等于 0,则冻结 `patch_embed` 模块的所有参数;如果 `frozen_stages` 大于等于 1,则冻结 `pos_drop` 模块以及前 `frozen_stages` 个 `layers` 模块的所有参数。在冻结之前,需要将相应的模块设置为 `eval` 模式,以便在冻结之后仍然能够保持参数不变。此外,需要将 `param.requires_grad` 设置为 `False`,以禁用梯度计算。
self.layers_strcuture = layers_strcuture self.layers_num = len(layers_strcuture) self.param_layers_num = self.layers_num - 1
这段代码的作用是将输入的神经网络层结构存储在实例变量 `self.layers_strcuture` 中,并计算出神经网络的总层数并存储在实例变量 `self.layers_num` 中。同时,由于输入的层结构中包含输入层,因此需要将其排除在计算参数层数的范围之外,所以将总层数减去1并存储在实例变量 `self.param_layers_num` 中。
相关推荐
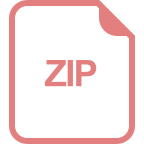
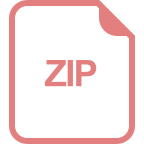













