1、使用继承Thread类的方式创建一个名为 SumThread的类,重写的run方法需要实现求规定区间的累加和。即:第一个线程从1加到10,第二个线程从11加到20,……,第十个线程从91加到100。 提示: 可通过获取当前线程的ID号,对ID号进行转换,用作求计算线程累加和的起始值; 需定义一个静态的全局数组sum[],存放第1到第10个线程各自求出的累加和。 2、在主程序中完成:将静态全局数组sum的各个元素的值相加并输出结果。 提示: 在启动10个线程之后,主线程需要休眠一段时间,等待各线程完成累加和的计算,然后再把各线程
时间: 2024-03-17 19:46:42 浏览: 68
计算出的累加和相加输出。
代码如下:
```
public class SumThread extends Thread {
private int start; // 起始值
private int end; // 结束值
public SumThread(int start, int end) {
this.start = start;
this.end = end;
}
@Override
public void run() {
int threadId = (int) Thread.currentThread().getId(); // 获取当前线程的ID号
int sum = 0;
for (int i = start; i <= end; i++) {
sum += i;
}
SumThread.sum[threadId - 1] = sum; // 将计算出的累加和存入静态全局数组sum中
}
public static int[] sum = new int[10]; // 静态全局数组sum,存放第1到第10个线程各自求出的累加和
}
public class Main {
public static void main(String[] args) throws InterruptedException {
SumThread[] threads = new SumThread[10]; // 创建10个线程
for (int i = 0; i < 10; i++) {
threads[i] = new SumThread(i * 10 + 1, (i + 1) * 10); // 每个线程计算的区间
threads[i].start(); // 启动线程
}
Thread.sleep(1000); // 主线程休眠1秒钟,等待各线程完成累加和的计算
int totalSum = 0;
for (int i = 0; i < 10; i++) {
totalSum += SumThread.sum[i]; // 将各线程计算出的累加和相加
}
System.out.println("总和为:" + totalSum);
}
}
```
阅读全文
相关推荐
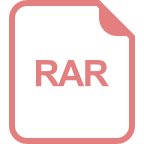
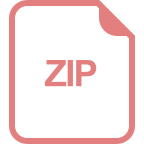
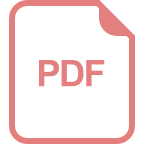
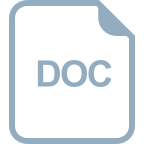
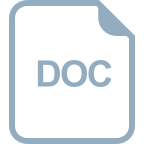
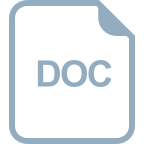
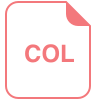
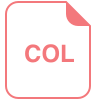
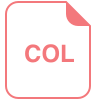
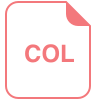
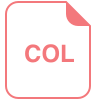
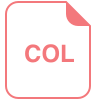
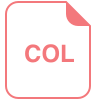
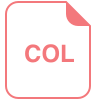



