opencv车牌识别参考代码
时间: 2023-08-25 16:04:28 浏览: 43
这里提供一个基于OpenCV的车牌识别参考代码,包含车牌定位、字符分割和字符识别三个部分:
```
import cv2
import numpy as np
import pytesseract
# 车牌定位
def locate_car_plate(image):
# 将图像转换为灰度图
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 运用高斯滤波平滑图像
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# 进行图像二值化
thresh = cv2.threshold(blur, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)[1]
# 找到图像中所有的轮廓
contours, hierarchy = cv2.findContours(thresh.copy(), cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 找到所有矩形轮廓
rects = [cv2.boundingRect(contour) for contour in contours]
# 筛选出符合条件的矩形轮廓
candidates = []
for rect in rects:
x, y, w, h = rect
if 0.8 <= w / h <= 4 and 1000 <= w * h <= 8000:
candidates.append(rect)
# 找到最大的矩形轮廓作为车牌区域
car_plate = image.copy()
if candidates:
car_plate_rect = max(candidates, key=lambda x: x[2] * x[3])
x, y, w, h = car_plate_rect
car_plate = image[y:y + h, x:x + w]
return car_plate
# 字符分割
def segment_characters(image):
# 将图像转换为灰度图
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 进行图像二值化
thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)[1]
# 找到图像中所有的轮廓
contours, hierarchy = cv2.findContours(thresh.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 找到所有矩形轮廓
rects = [cv2.boundingRect(contour) for contour in contours]
# 筛选出符合条件的矩形轮廓
chars = []
for rect in rects:
x, y, w, h = rect
if 5 <= w <= 200 and 10 <= h <= 200 and 0.35 <= w / h <= 1.5:
chars.append(rect)
# 将字符按照位置排序
chars = sorted(chars, key=lambda x: x[0])
return chars
# 字符识别
def recognize_characters(image, chars):
# 将图像转换为灰度图
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 进行图像二值化
thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)[1]
# 将字符按照位置排序
chars = sorted(chars, key=lambda x: x[0])
# 识别每个字符
result = ''
for rect in chars:
x, y, w, h = rect
char = thresh[y:y + h, x:x + w]
char = cv2.resize(char, (36, 36))
char = cv2.copyMakeBorder(char, 2, 2, 2, 2, cv2.BORDER_CONSTANT, value=0)
char = cv2.cvtColor(char, cv2.COLOR_GRAY2BGR)
char = cv2.resize(char, (36, 36), interpolation=cv2.INTER_AREA)
char = cv2.dnn.blobFromImage(char, scalefactor=1 / 255.0, size=(36, 36), mean=(0, 0, 0), swapRB=True, crop=False)
net.setInput(char)
preds = net.forward()
index = np.argmax(preds)
result += chars_dict[index]
return result
# 加载字符识别模型
net = cv2.dnn.readNet('char_recognition_model.weights', 'char_recognition_model.cfg')
# 字符编码表
chars_dict = {0: '0', 1: '1', 2: '2', 3: '3', 4: '4', 5: '5', 6: '6', 7: '7', 8: '8', 9: '9', 10: 'A', 11: 'B',
12: 'C', 13: 'D', 14: 'E', 15: 'F', 16: 'G', 17: 'H', 18: 'I', 19: 'J', 20: 'K', 21: 'L', 22: 'M',
23: 'N', 24: 'O', 25: 'P', 26: 'Q', 27: 'R', 28: 'S', 29: 'T', 30: 'U', 31: 'V', 32: 'W', 33: 'X',
34: 'Y', 35: 'Z'}
# 读取车辆图片
image = cv2.imread('car.jpg')
# 车牌定位
car_plate = locate_car_plate(image)
# 字符分割
chars = segment_characters(car_plate)
# 字符识别
result = recognize_characters(car_plate, chars)
# 显示结果
print(result)
# 使用pytesseract进行OCR识别
text = pytesseract.image_to_string(car_plate, lang='chi_sim')
print(text)
```
在该代码中,首先进行车牌定位,然后对车牌进行字符分割,最后使用深度学习模型进行字符识别。在字符识别部分,我们将每个字符resize到36x36,然后作为输入传入深度学习模型进行预测,得到最终的车牌字符识别结果。除了使用深度学习模型进行字符识别外,我们还可以使用OCR库pytesseract进行车牌识别。
相关推荐
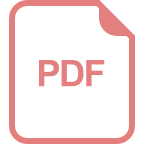














