用python语言编写一个计算两个经纬度之间距离的代码
时间: 2023-07-23 21:21:44 浏览: 67
可以使用haversine公式来计算两个经纬度之间的距离,以下是用Python实现的代码:
```python
from math import radians, sin, cos, sqrt, atan2
def distance(lat1, lon1, lat2, lon2):
R = 6371 # 地球半径,单位为公里
lat1, lon1, lat2, lon2 = map(radians, [lat1, lon1, lat2, lon2]) # 将经纬度转换为弧度
dlat = lat2 - lat1
dlon = lon2 - lon1
a = sin(dlat/2)**2 + cos(lat1) * cos(lat2) * sin(dlon/2)**2
c = 2 * atan2(sqrt(a), sqrt(1-a))
distance = R * c # 计算出两个经纬度之间的距离,单位为公里
return distance
```
其中,lat1和lon1表示第一个点的经纬度,lat2和lon2表示第二个点的经纬度,返回值为两点之间的距离,单位为公里。
相关问题
我需要编写一段代码,用来计算两个经纬度之间的距离
您可以使用 Haversine 公式来计算两个经纬度之间的距离。以下是一个示例 Python 代码:
```python
import math
def distance(lat1, lon1, lat2, lon2):
R = 6371 # 地球半径,单位为千米
dlat = math.radians(lat2 - lat1)
dlon = math.radians(lon2 - lon1)
a = math.sin(dlat / 2) ** 2 + math.cos(math.radians(lat1)) * math.cos(math.radians(lat2)) * math.sin(dlon / 2) ** 2
c = 2 * math.atan2(math.sqrt(a), math.sqrt(1 - a))
return R * c
```
其中,`lat1` 和 `lon1` 是第一个经纬度,`lat2` 和 `lon2` 是第二个经纬度。函数返回值为两个经纬度之间的距离,单位为千米。
用python语言编写代码 已知两点的经纬度,绘制连接两点的一段曲线
可以使用Matplotlib库中的plot函数,具体代码如下:
import matplotlib.pyplot as plt
x1,y1 = 第一个点的经纬度
x2,y2 = 第二个点的经纬度
plt.plot([x1,x2],[y1,y2])
plt.show()
相关推荐
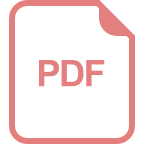
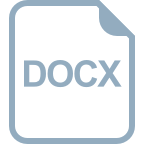












