用Android Studio做一个天气预报app详细代码
时间: 2023-11-15 10:44:31 浏览: 183
这是一个简单的天气预报app的示例代码,使用了OpenWeatherMap的API获取天气数据。你需要先注册OpenWeatherMap账号获取API Key。
1. 布局文件
在`res/layout`目录下创建一个名为`activity_main.xml`的布局文件,添加以下代码:
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/city_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="Loading..." />
<TextView
android:id="@+id/temp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="20sp"
android:text="Loading..." />
<TextView
android:id="@+id/weather_desc"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="20sp"
android:text="Loading..." />
</LinearLayout>
```
2. Java代码
在`app/src/main/java`目录下创建一个名为`MainActivity.java`的Java类,添加以下代码:
```java
public class MainActivity extends AppCompatActivity {
private static final String API_KEY = "YOUR_API_KEY"; // 替换成你的API Key
private static final String API_URL = "https://api.openweathermap.org/data/2.5/weather?q=%s&appid=%s&units=metric";
private TextView cityNameTextView;
private TextView tempTextView;
private TextView weatherDescTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
cityNameTextView = findViewById(R.id.city_name);
tempTextView = findViewById(R.id.temp);
weatherDescTextView = findViewById(R.id.weather_desc);
String city = "beijing"; // 替换成你要查询的城市
String apiUrl = String.format(API_URL, city, API_KEY);
JsonObjectRequest request = new JsonObjectRequest(Request.Method.GET, apiUrl, null,
response -> {
try {
JSONObject main = response.getJSONObject("main");
JSONArray weatherArray = response.getJSONArray("weather");
JSONObject weather = weatherArray.getJSONObject(0);
String cityName = response.getString("name");
String temp = main.getString("temp") + " °C";
String weatherDesc = weather.getString("description");
cityNameTextView.setText(cityName);
tempTextView.setText(temp);
weatherDescTextView.setText(weatherDesc);
} catch (JSONException e) {
e.printStackTrace();
}
}, error -> {
Toast.makeText(this, "Failed to fetch weather data", Toast.LENGTH_SHORT).show();
Log.e("MainActivity", "Failed to fetch weather data", error);
});
RequestQueue queue = Volley.newRequestQueue(this);
queue.add(request);
}
}
```
注意替换代码中的`YOUR_API_KEY`和`city`变量。
3. 运行app
运行app并输入查询的城市名称,即可获取天气数据。
阅读全文
相关推荐
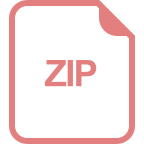




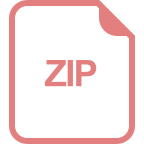
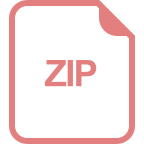
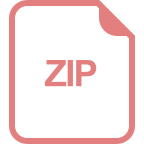
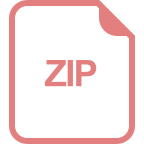
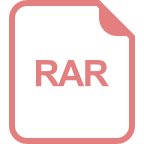
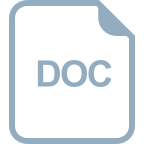





