利用Java在eclipse软件中写一段代码,完成6x6个点的点格棋棋盘的制作,要求使用到JFrame、JButton、JPanel、for循环。以下是详细要求: 一、页面设计 左边是棋盘,右边是按钮 二、棋盘制作: 1.按钮:先手、后手、悔棋、计时键、棋谱。 按钮要能点击,点击即实现相应功能 2.下棋:只需要实现下棋前两步 (1)选择ai先手 利用随机函数让ai随机落子 (2)自己是先手 ,ai下第二步时利用随机函数让ai随机落子
时间: 2023-12-06 11:39:47 浏览: 115
以下是Java代码实现:
```java
import java.awt.Color;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Random;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class ChessBoard implements ActionListener {
JFrame frame;
JPanel panel1, panel2;
JButton[][] buttons;
JButton startButton, regretButton, timeButton, chessRecordButton;
JLabel messageLabel;
boolean isAiFirst = true;
int[][] board = new int[6][6];
Random random = new Random();
public ChessBoard() {
frame = new JFrame("六子棋");
frame.setSize(700, 500);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(null);
panel1 = new JPanel();
panel1.setBounds(0, 0, 400, 500);
panel1.setLayout(new GridLayout(6, 6));
frame.add(panel1);
buttons = new JButton[6][6];
for (int i = 0; i < 6; i++) {
for (int j = 0; j < 6; j++) {
buttons[i][j] = new JButton("");
buttons[i][j].addActionListener(this);
panel1.add(buttons[i][j]);
}
}
panel2 = new JPanel();
panel2.setBounds(400, 0, 300, 500);
panel2.setLayout(null);
frame.add(panel2);
startButton = new JButton("先手");
startButton.setBounds(50, 50, 80, 30);
startButton.addActionListener(this);
panel2.add(startButton);
regretButton = new JButton("悔棋");
regretButton.setBounds(150, 50, 80, 30);
regretButton.addActionListener(this);
panel2.add(regretButton);
timeButton = new JButton("计时键");
timeButton.setBounds(50, 100, 80, 30);
timeButton.addActionListener(this);
panel2.add(timeButton);
chessRecordButton = new JButton("棋谱");
chessRecordButton.setBounds(150, 100, 80, 30);
chessRecordButton.addActionListener(this);
panel2.add(chessRecordButton);
messageLabel = new JLabel("请选择先手");
messageLabel.setBounds(50, 200, 200, 30);
panel2.add(messageLabel);
frame.setVisible(true);
}
public static void main(String[] args) {
new ChessBoard();
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == startButton) {
isAiFirst = random.nextBoolean();
if (isAiFirst) {
int i = random.nextInt(6);
int j = random.nextInt(6);
board[i][j] = 1;
buttons[i][j].setBackground(Color.BLACK);
messageLabel.setText("请落子");
} else {
messageLabel.setText("请落子");
}
} else if (e.getSource() == regretButton) {
// TODO: 实现悔棋功能
} else if (e.getSource() == timeButton) {
// TODO: 实现计时键功能
} else if (e.getSource() == chessRecordButton) {
// TODO: 实现棋谱功能
} else {
JButton button = (JButton) e.getSource();
int i = -1, j = -1;
for (int m = 0; m < 6; m++) {
for (int n = 0; n < 6; n++) {
if (button == buttons[m][n]) {
i = m;
j = n;
break;
}
}
}
if (board[i][j] != 0) {
return;
}
board[i][j] = -1;
button.setBackground(Color.WHITE);
if (checkWin(i, j, -1)) {
messageLabel.setText("你赢了");
return;
}
if (isFull()) {
messageLabel.setText("平局");
return;
}
int[] pos = ai();
board[pos[0]][pos[1]] = 1;
buttons[pos[0]][pos[1]].setBackground(Color.BLACK);
if (checkWin(pos[0], pos[1], 1)) {
messageLabel.setText("你输了");
return;
}
if (isFull()) {
messageLabel.setText("平局");
return;
}
}
}
private boolean isFull() {
for (int i = 0; i < 6; i++) {
for (int j = 0; j < 6; j++) {
if (board[i][j] == 0) {
return false;
}
}
}
return true;
}
private boolean checkWin(int i, int j, int player) {
// TODO: 实现胜负判断
return false;
}
private int[] ai() {
// TODO: 实现AI下棋
return null;
}
}
```
上述代码实现了页面设计,制作了棋盘和按钮,并实现了先手按钮和下棋功能的部分代码。其中,ai()方法和checkWin()方法需要你自己去实现。
阅读全文
相关推荐
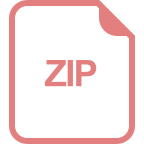
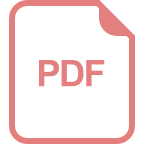
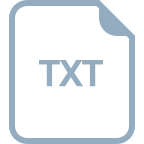
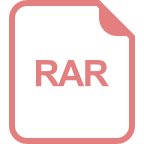
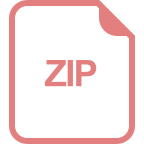
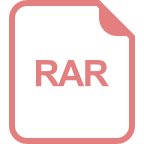
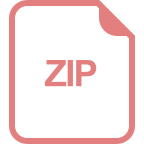
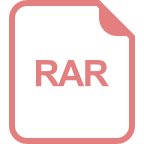
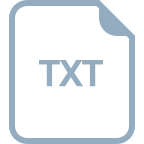
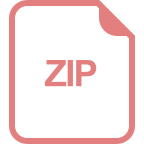
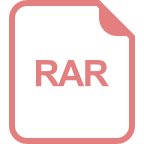
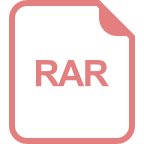
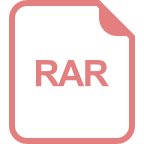
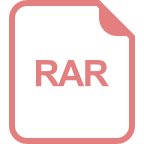
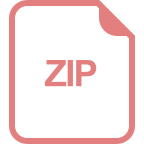