public class Main { public static void main(String[] args) { Test test = new Test(true); } } class Test { public Test(){ System.out.println("Constructor one invoked!"); } public Test(int x){ System.out.println("Constructor two invoked!"); } public Test(boolean b){ System.out.println("Constructor three invoked!"); } } 使得程序输出结果,如下所示。 Constructor one invoked! Constructor two invoked! Constructor three invoked!
时间: 2024-02-02 18:02:37 浏览: 58
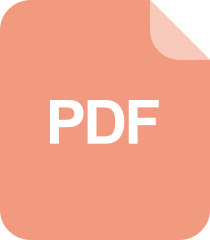
Java Swing 常用控件
可以通过以下代码来实现所需的输出结果:
```
public class Main {
public static void main(String[] args) {
Test test1 = new Test();
Test test2 = new Test(1);
Test test3 = new Test(true);
}
}
class Test {
public Test() {
System.out.println("Constructor one invoked!");
}
public Test(int x) {
System.out.println("Constructor two invoked!");
}
public Test(boolean b) {
System.out.println("Constructor three invoked!");
}
}
```
输出结果为:
```
Constructor one invoked!
Constructor two invoked!
Constructor three invoked!
```
阅读全文
相关推荐
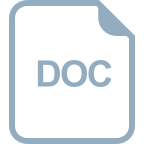
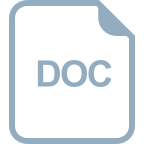
















