如何用java实现在吃货和厨师案例中,假设这个桌子可以存储10个汉堡,只要桌子上不够10个汉堡厨师就会一直生产,只要桌子上的汉堡没有吃光 那么吃货就会一直吃, 桌子上没有汉堡了吃货就会等待,桌子上汉堡满10个了,厨师就会等待
时间: 2023-05-11 13:04:05 浏览: 102
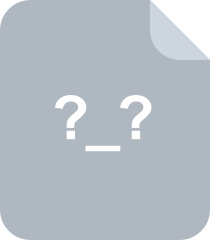
哲学家进餐问题,JAVA多线程实现

可以使用Java中的多线程来实现这个场景。首先定义一个桌子类,其中包含一个汉堡数量的变量和对应的生产和消费方法。然后定义一个厨师类和一个吃货类,分别继承自线程类,实现对桌子的生产和消费操作。具体实现代码如下:
```java
class Table {
private int burgerNum = 0;
public synchronized void produce() {
while (burgerNum >= 10) {
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
burgerNum++;
System.out.println("厨师生产了一个汉堡,当前桌子上有" + burgerNum + "个汉堡");
notifyAll();
}
public synchronized void consume() {
while (burgerNum <= 0) {
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
burgerNum--;
System.out.println("吃货吃了一个汉堡,当前桌子上有" + burgerNum + "个汉堡");
notifyAll();
}
}
class Chef extends Thread {
private Table table;
public Chef(Table table) {
this.table = table;
}
@Override
public void run() {
while (true) {
table.produce();
}
}
}
class Eater extends Thread {
private Table table;
public Eater(Table table) {
this.table = table;
}
@Override
public void run() {
while (true) {
table.consume();
}
}
}
public class Main {
public static void main(String[] args) {
Table table = new Table();
Chef chef = new Chef(table);
Eater eater = new Eater(table);
chef.start();
eater.start();
}
}
```
在这个实现中,桌子类中的生产和消费方法都使用了synchronized关键字来保证线程安全。厨师和吃货类分别继承自线程类,并在run方法中不断地调用桌子类的生产和消费方法。在主函数中创建桌子、厨师和吃货对象,并启动线程。这样就可以实现一个简单的生产者消费者模型。
阅读全文
相关推荐
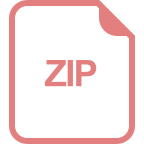
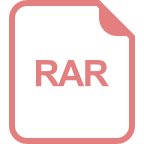
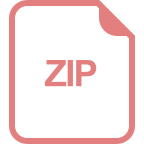
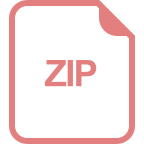
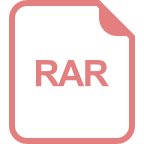
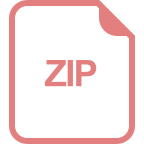
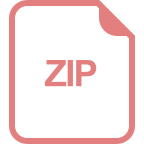
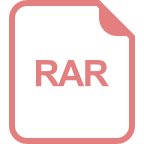
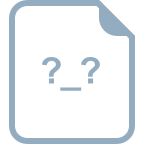
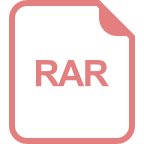
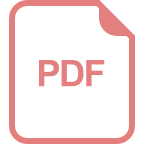
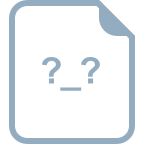
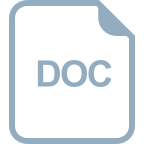
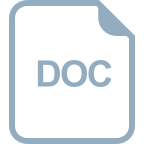
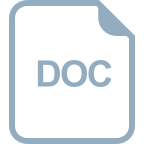