python调用yolov5实现实时自动瞄准
时间: 2023-08-12 15:57:32 浏览: 160
要使用YOLOv5实现实时自动瞄准功能,您可以按照以下步骤进行操作:
1. 安装必要的依赖库:
```shell
pip install opencv-python
pip install torch torchvision
pip install yolov5
```
2. 下载YOLOv5权重文件:
您可以从YOLOv5的GitHub仓库(https://github.com/ultralytics/yolov5)下载预训练的权重文件,并将其保存在与您的Python脚本相同的目录下。
3. 编写Python脚本:
```python
import cv2
import torch
import numpy as np
from yolov5.models.experimental import attempt_load
from yolov5.utils.general import non_max_suppression, scale_coords
def auto_aim(image):
# 加载YOLOv5模型
weights = 'yolov5s.pt' # 根据您下载的权重文件进行更改
model = attempt_load(weights, map_location=torch.device('cpu'))
model.eval()
# 图像预处理
img = letterbox(image, new_shape=640)[0]
img = img[:, :, ::-1].transpose(2, 0, 1) # BGR to RGB
img = np.ascontiguousarray(img)
img = torch.from_numpy(img).unsqueeze(0)
img /= 255.0
# 目标检测
with torch.no_grad():
outputs = model(img)
outputs = non_max_suppression(outputs, conf_thres=0.3, iou_thres=0.5)
# 解析检测结果
if outputs[0] is not None:
detections = outputs[0]
detections[:, :4] = scale_coords(img.shape[2:], detections[:, :4], image.shape).round()
# 取第一个检测结果的中心坐标
x_center = (detections[0][0] + detections[0][2]) / 2
y_center = (detections[0][1] + detections[0][3]) / 2
# 返回中心坐标
return int(x_center), int(y_center)
return None
def main():
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取视频帧
ret, frame = cap.read()
# 自动瞄准
target = auto_aim(frame)
if target is not None:
x, y = target
print(f"自动瞄准目标坐标:({x}, {y})")
# 在图像上绘制目标点
cv2.circle(frame, (x, y), 5, (0, 255, 0), -1)
# 显示图像
cv2.imshow("Frame", frame)
# 按下q键退出循环
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头并关闭窗口
cap.release()
cv2.destroyAllWindows()
if __name__ == "__main__":
main()
```
在上述代码中,我们使用OpenCV打开了摄像头,并读取实时视频帧。然后,我们对每一帧图像进行自动瞄准,获取目标的中心坐标,并在图像上绘制目标点。最后,我们通过按下`q`键来退出循环,释放摄像头并关闭窗口。
请注意,您需要将代码中的`yolov5s.pt`替换为您下载的YOLOv5权重文件的路径。另外,您可能需要根据您的实际情况调整模型权重文件和阈值参数。
阅读全文
相关推荐
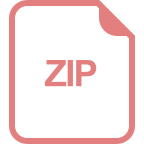
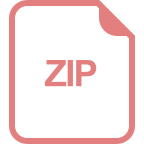
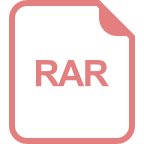
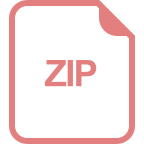
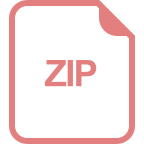
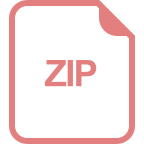
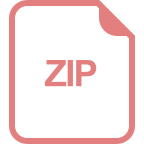
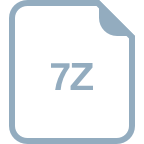
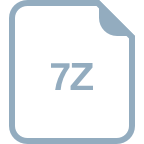
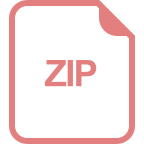
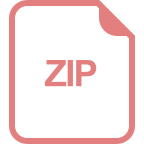
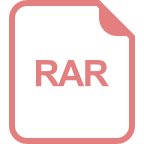
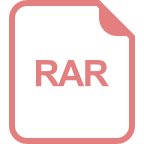
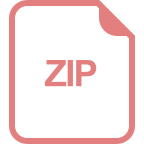
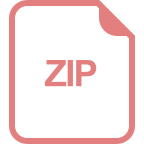
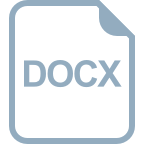
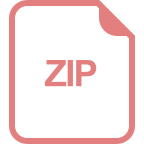
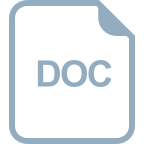