给我写一个贪吃蛇,用c++
时间: 2023-07-04 19:28:22 浏览: 48
好的,以下是一个简单的贪吃蛇游戏的C++实现:
```c++
#include<iostream>
#include<conio.h>
#include<windows.h>
using namespace std;
//定义游戏区域大小
const int width = 20;
const int height = 20;
//定义蛇和食物的坐标
int snakeX[100], snakeY[100], foodX, foodY;
//定义蛇的长度和分数
int snakeLength = 1;
int score = 0;
//定义方向
enum eDirection{STOP = 0, LEFT, RIGHT, UP, DOWN};
eDirection dir;
//初始化游戏
void init()
{
//初始化蛇的位置在游戏区域中央
snakeX[0] = width / 2;
snakeY[0] = height / 2;
//初始化食物位置
foodX = rand() % width;
foodY = rand() % height;
//初始化方向
dir = STOP;
}
//绘制游戏区域
void draw()
{
system("cls"); //清屏
//绘制上边界
for (int i = 0; i < width + 2; i++)
cout << "#";
cout << endl;
//绘制游戏区域
for (int i = 0; i < height; i++)
{
for (int j = 0; j < width; j++)
{
if (j == 0)
cout << "#";
if (i == snakeY[0] && j == snakeX[0])
cout << "O"; //蛇头
else if (i == foodY && j == foodX)
cout << "F"; //食物
else
{
bool print = false;
for (int k = 1; k < snakeLength; k++)
{
if (snakeX[k] == j && snakeY[k] == i)
{
cout << "o"; //蛇身
print = true;
}
}
if (!print)
cout << " ";
}
if (j == width - 1)
cout << "#";
}
cout << endl;
}
//绘制下边界
for (int i = 0; i < width + 2; i++)
cout << "#";
cout << endl;
//显示分数
cout << "Score:" << score << endl;
}
//获取键盘输入
void input()
{
if (_kbhit())
{
switch (_getch())
{
case 'a':
dir = LEFT;
break;
case 'd':
dir = RIGHT;
break;
case 'w':
dir = UP;
break;
case 's':
dir = DOWN;
break;
case 'x':
dir = STOP;
break;
}
}
}
//游戏逻辑
void logic()
{
//移动蛇身
for (int i = snakeLength - 1; i > 0; i--)
{
snakeX[i] = snakeX[i - 1];
snakeY[i] = snakeY[i - 1];
}
//根据方向移动蛇头
switch (dir)
{
case LEFT:
snakeX[0]--;
break;
case RIGHT:
snakeX[0]++;
break;
case UP:
snakeY[0]--;
break;
case DOWN:
snakeY[0]++;
break;
}
//判断是否吃到食物
if (snakeX[0] == foodX && snakeY[0] == foodY)
{
score += 10;
snakeLength++;
foodX = rand() % width;
foodY = rand() % height;
}
//判断是否撞墙或撞到自己
if (snakeX[0] < 0 || snakeX[0] >= width || snakeY[0] < 0 || snakeY[0] >= height)
{
cout << "Game Over!" << endl;
exit(0);
}
for (int i = 1; i < snakeLength; i++)
{
if (snakeX[i] == snakeX[0] && snakeY[i] == snakeY[0])
{
cout << "Game Over!" << endl;
exit(0);
}
}
}
//主程序
int main()
{
init(); //初始化游戏
while (true)
{
draw(); //绘制游戏
input(); //获取键盘输入
logic(); //游戏逻辑
Sleep(50); //延时
}
return 0;
}
```
注意:这只是一个简单的实现,还有很多可以改进的地方,比如增加难度、增加音效、增加暂停功能等。
相关推荐
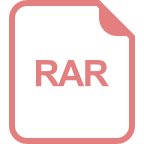









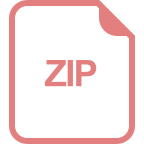