用C++写一个贪吃蛇
时间: 2023-08-31 08:06:24 浏览: 93
好的,下面是一个简单的贪吃蛇游戏的C++代码:
```cpp
#include <iostream>
#include <conio.h> // for _getch()
#include <windows.h> // for Sleep()
using namespace std;
// 游戏区域大小
const int width = 20;
const int height = 20;
// 蛇的初始长度
const int initLength = 3;
// 蛇和食物的标记
const char snakeMark = '*';
const char foodMark = '@';
// 蛇的移动方向
enum Direction
{
STOP = 0,
UP,
DOWN,
LEFT,
RIGHT
};
// 游戏状态
bool gameOver = false;
// 蛇的当前位置、长度和方向
int snakeX[width * height], snakeY[width * height];
int snakeLength = initLength;
Direction direction = STOP;
// 食物的位置
int foodX, foodY;
// 游戏得分
int score = 0;
// 初始化游戏
void Init()
{
// 初始化蛇的位置
snakeX[0] = width / 2;
snakeY[0] = height / 2;
for (int i = 1; i < snakeLength; ++i)
{
snakeX[i] = snakeX[i - 1] + 1;
snakeY[i] = snakeY[i - 1];
}
// 随机生成食物
srand((unsigned)time(NULL));
foodX = rand() % (width - 2) + 1;
foodY = rand() % (height - 2) + 1;
// 初始化游戏状态和得分
gameOver = false;
score = 0;
}
// 绘制游戏画面
void Draw()
{
system("cls"); // 清屏
// 绘制游戏区域
for (int i = 0; i < width + 2; ++i)
cout << '#';
cout << endl;
for (int i = 0; i < height; ++i)
{
cout << '#';
for (int j = 0; j < width; ++j)
{
if (i == foodY && j == foodX)
cout << foodMark; // 绘制食物
else if (i == snakeY[0] && j == snakeX[0])
cout << snakeMark; // 绘制蛇头
else
{
bool body = false;
for (int k = 1; k < snakeLength; ++k)
{
if (i == snakeY[k] && j == snakeX[k])
{
cout << snakeMark; // 绘制蛇身
body = true;
break;
}
}
if (!body)
cout << ' '; // 绘制空格
}
}
cout << '#' << endl;
}
for (int i = 0; i < width + 2; ++i)
cout << '#';
cout << endl;
// 显示得分
cout << "Score: " << score << endl;
}
// 处理用户输入
void Input()
{
if (_kbhit()) // 检测是否有键盘输入
{
char ch = _getch();
switch (ch)
{
case 'w':
case 'W':
if (direction != DOWN)
direction = UP;
break;
case 's':
case 'S':
if (direction != UP)
direction = DOWN;
break;
case 'a':
case 'A':
if (direction != RIGHT)
direction = LEFT;
break;
case 'd':
case 'D':
if (direction != LEFT)
direction = RIGHT;
break;
case 'x':
case 'X':
gameOver = true;
break;
}
}
}
// 更新游戏状态
void Update()
{
// 移动蛇的身体
for (int i = snakeLength - 1; i > 0; --i)
{
snakeX[i] = snakeX[i - 1];
snakeY[i] = snakeY[i - 1];
}
// 移动蛇的头部
switch (direction)
{
case UP:
--snakeY[0];
break;
case DOWN:
++snakeY[0];
break;
case LEFT:
--snakeX[0];
break;
case RIGHT:
++snakeX[0];
break;
}
// 检测是否吃到食物
if (snakeX[0] == foodX && snakeY[0] == foodY)
{
snakeLength++;
score += 10;
// 生成新的食物
foodX = rand() % (width - 2) + 1;
foodY = rand() % (height - 2) + 1;
}
// 检测是否撞墙或撞到自己
if (snakeX[0] == 0 || snakeX[0] == width - 1 || snakeY[0] == 0 || snakeY[0] == height - 1)
gameOver = true;
for (int i = 1; i < snakeLength; ++i)
{
if (snakeX[0] == snakeX[i] && snakeY[0] == snakeY[i])
gameOver = true;
}
}
int main()
{
Init();
while (!gameOver)
{
Draw();
Input();
Update();
Sleep(100); // 暂停一段时间,控制游戏速度
}
cout << "Game Over!" << endl;
return 0;
}
```
注意:这个代码只是一个简单的贪吃蛇游戏,还有很多可以改进的地方,比如增加难度、增加音效等。
阅读全文
相关推荐


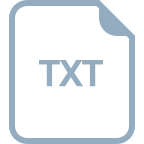
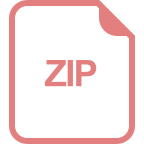
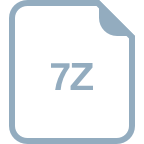