编写代码实现词法分析器
时间: 2023-09-26 07:09:14 浏览: 41
好的,下面是一个简单的词法分析器的代码实现示例,它可以识别出数字、标识符、运算符、括号和分号等符号:
```python
import re
# 定义关键字列表
keywords = ['if', 'else', 'while', 'for', 'int', 'float', 'double']
# 定义运算符列表
operators = ['+', '-', '*', '/', '=', '>', '<', '>=', '<=', '==', '!=']
# 定义符号列表
symbols = ['(', ')', '{', '}', '[', ']', ';', ',']
# 定义数字匹配正则表达式
num_pattern = re.compile(r'^\d+(\.\d+)?$')
# 定义标识符匹配正则表达式
id_pattern = re.compile(r'^[a-zA-Z_]\w*$')
# 定义源代码字符串
source_code = '''
int main() {
int a = 10;
int b = 20;
int c = a + b;
if (c > 30) {
printf("Result: %d\\n", c);
} else {
printf("Result is too small.\\n");
}
return 0;
}
'''
# 分词函数
def tokenize(code):
tokens = []
i = 0
while i < len(code):
# 跳过空格和换行符
if code[i].isspace():
i += 1
continue
# 匹配数字
if num_pattern.match(code[i:]):
match = num_pattern.match(code[i:])
token = match.group()
tokens.append(('NUMBER', token))
i += len(token)
continue
# 匹配标识符或关键字
if id_pattern.match(code[i:]):
match = id_pattern.match(code[i:])
token = match.group()
if token in keywords:
tokens.append(('KEYWORD', token))
else:
tokens.append(('ID', token))
i += len(token)
continue
# 匹配运算符
if code[i] in operators:
tokens.append(('OPERATOR', code[i]))
i += 1
continue
# 匹配符号
if code[i] in symbols:
tokens.append(('SYMBOL', code[i]))
i += 1
continue
# 无法匹配的字符
tokens.append(('UNKNOWN', code[i]))
i += 1
return tokens
# 测试分词函数
tokens = tokenize(source_code)
for token in tokens:
print(token)
```
这个示例代码使用了 Python 语言,通过正则表达式匹配数字和标识符,并使用循环遍历源代码,逐个字符地进行匹配。最终,它会输出每个标记的类型和值。当然,在实际应用中,词法分析器的实现会更加复杂,需要考虑各种特殊情况和错误处理。
相关推荐
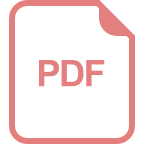
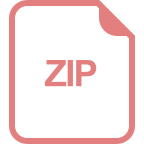
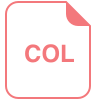
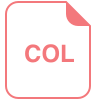
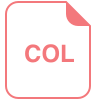
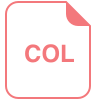
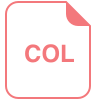









