java开发扫雷游戏的源代码是什么
时间: 2023-05-17 10:05:40 浏览: 147
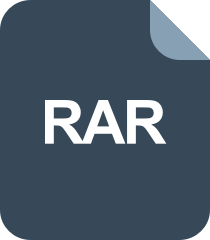
Java的扫雷游戏的源代码
非常感谢您的提问!以下是一个简单的 Java 扫雷游戏的源代码,希望能够帮到您:
```java
import java.util.Random;
import java.util.Scanner;
public class Minesweeper {
private static final int EASY_ROWS = 9;
private static final int EASY_COLS = 9;
private static final int EASY_MINES = 10;
private static final int MEDIUM_ROWS = 16;
private static final int MEDIUM_COLS = 16;
private static final int MEDIUM_MINES = 40;
private static final int HARD_ROWS = 16;
private static final int HARD_COLS = 30;
private static final int HARD_MINES = 99;
private static final int COVERED = -1;
private static final int MINE = -2;
private static final int[] ROW_OFFSETS = {-1, -1, -1, 0, 0, 1, 1, 1};
private static final int[] COL_OFFSETS = {-1, 0, 1, -1, 1, -1, 0, 1};
private int rows;
private int cols;
private int mines;
private int[][] board;
private boolean[][] revealed;
public Minesweeper(int rows, int cols, int mines) {
this.rows = rows;
this.cols = cols;
this.mines = mines;
this.board = new int[rows][cols];
this.revealed = new boolean[rows][cols];
initializeBoard();
}
private void initializeBoard() {
Random random = new Random();
int remainingMines = mines;
while (remainingMines > 0) {
int row = random.nextInt(rows);
int col = random.nextInt(cols);
if (board[row][col] != MINE) {
board[row][col] = MINE;
remainingMines--;
}
}
for (int row = 0; row < rows; row++) {
for (int col = 0; col < cols; col++) {
if (board[row][col] != MINE) {
int count = 0;
for (int i = 0; i < ROW_OFFSETS.length; i++) {
int newRow = row + ROW_OFFSETS[i];
int newCol = col + COL_OFFSETS[i];
if (newRow >= 0 && newRow < rows && newCol >= 0 && newCol < cols && board[newRow][newCol] == MINE) {
count++;
}
}
board[row][col] = count;
}
}
}
}
public void play() {
Scanner scanner = new Scanner(System.in);
while (true) {
printBoard();
System.out.print("Enter row and column (e.g. 3 4): ");
int row = scanner.nextInt() - 1;
int col = scanner.nextInt() - 1;
if (row < 0 || row >= rows || col < 0 || col >= cols) {
System.out.println("Invalid row or column!");
continue;
}
if (board[row][col] == MINE) {
System.out.println("Game over!");
printBoard(true);
break;
}
reveal(row, col);
if (isWin()) {
System.out.println("You win!");
printBoard(true);
break;
}
}
}
private void reveal(int row, int col) {
if (revealed[row][col]) {
return;
}
revealed[row][col] = true;
if (board[row][col] == 0) {
for (int i = 0; i < ROW_OFFSETS.length; i++) {
int newRow = row + ROW_OFFSETS[i];
int newCol = col + COL_OFFSETS[i];
if (newRow >= 0 && newRow < rows && newCol >= 0 && newCol < cols) {
reveal(newRow, newCol);
}
}
}
}
private boolean isWin() {
int count = 0;
for (int row = 0; row < rows; row++) {
for (int col = 0; col < cols; col++) {
if (board[row][col] != MINE && revealed[row][col]) {
count++;
}
}
}
return count == rows * cols - mines;
}
private void printBoard() {
printBoard(false);
}
private void printBoard(boolean revealMines) {
System.out.print(" ");
for (int col = 0; col < cols; col++) {
System.out.print(col + 1);
}
System.out.println();
System.out.print(" ");
for (int col = 0; col < cols; col++) {
System.out.print("-");
}
System.out.println();
for (int row = 0; row < rows; row++) {
System.out.print(row + 1 + "|");
for (int col = 0; col < cols; col++) {
if (revealed[row][col] || revealMines && board[row][col] == MINE) {
if (board[row][col] == MINE) {
System.out.print("*");
} else if (board[row][col] == 0) {
System.out.print(" ");
} else {
System.out.print(board[row][col]);
}
} else {
System.out.print("#");
}
}
System.out.println("|" + (row + 1));
}
System.out.print(" ");
for (int col = 0; col < cols; col++) {
System.out.print("-");
}
System.out.println();
System.out.print(" ");
for (int col = 0; col < cols; col++) {
System.out.print(col + 1);
}
System.out.println();
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Select difficulty level:");
System.out.println("1. Easy");
System.out.println("2. Medium");
System.out.println("3. Hard");
int choice = scanner.nextInt();
int rows, cols, mines;
switch (choice) {
case 1:
rows = EASY_ROWS;
cols = EASY_COLS;
mines = EASY_MINES;
break;
case 2:
rows = MEDIUM_ROWS;
cols = MEDIUM_COLS;
mines = MEDIUM_MINES;
break;
case 3:
rows = HARD_ROWS;
cols = HARD_COLS;
mines = HARD_MINES;
break;
default:
System.out.println("Invalid choice!");
return;
}
Minesweeper game = new Minesweeper(rows, cols, mines);
game.play();
}
}
```
希望这个源代码能够满足您的需求!
阅读全文
相关推荐
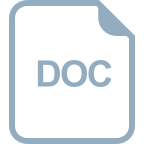
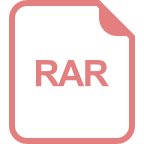
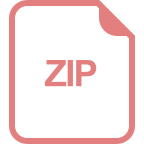
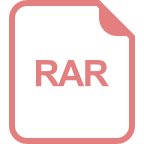
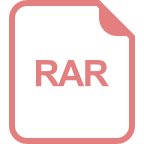
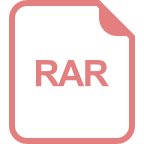
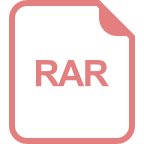
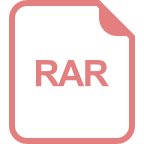
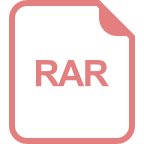
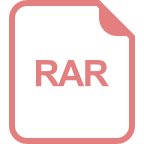
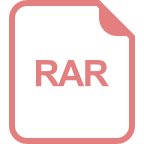
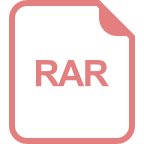
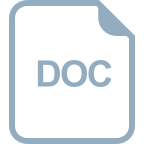
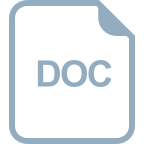
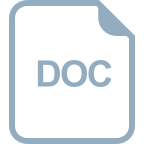
