vue结合three.js开发可视化项目实现一个动态立体的demo
时间: 2024-02-17 19:00:16 浏览: 128
好的,下面是一些指导,帮助您实现动态立体的demo。
1. 集成Three.js到Vue.js中
首先,您需要将Three.js集成到Vue.js中。您可以使用npm安装Three.js和Vue.js:
```
npm install three vue
```
然后在组件中导入Three.js:
```javascript
import * as THREE from 'three';
```
2. 创建一个Three.js场景
接下来,您需要在Vue.js应用程序中创建一个Three.js场景。您可以使用Vue.js的生命周期方法来初始化和更新Three.js场景。
```javascript
mounted() {
// 创建一个Three.js场景
this.scene = new THREE.Scene();
// 添加相机
this.camera = new THREE.PerspectiveCamera(45, window.innerWidth / window.innerHeight, 0.1, 1000);
// 添加渲染器
this.renderer = new THREE.WebGLRenderer({ antialias: true });
this.renderer.setSize(window.innerWidth, window.innerHeight);
this.renderer.setClearColor(0x000000, 1);
// 添加场景元素
this.addElements();
// 将渲染器添加到DOM中
this.$el.appendChild(this.renderer.domElement);
},
```
在这个例子中,我们添加了一个相机和一个渲染器。我们还调用了一个addElements()方法,用于添加场景元素。
3. 添加场景元素
接下来,您需要添加一些场景元素,例如立方体、球体、光源等。您可以使用Three.js提供的各种几何体和材质来创建这些元素。
```javascript
addElements() {
// 添加一个立方体
const geometry = new THREE.BoxGeometry(1, 1, 1);
const material = new THREE.MeshBasicMaterial({ color: 0xffffff });
this.cube = new THREE.Mesh(geometry, material);
this.scene.add(this.cube);
// 添加一个球体
const sphereGeometry = new THREE.SphereGeometry(0.5, 32, 32);
const sphereMaterial = new THREE.MeshBasicMaterial({ color: 0xff0000 });
this.sphere = new THREE.Mesh(sphereGeometry, sphereMaterial);
this.scene.add(this.sphere);
// 添加一个点光源
const light = new THREE.PointLight(0xffffff, 1, 100);
light.position.set(0, 0, 10);
this.scene.add(light);
},
```
在这个例子中,我们添加了一个立方体、一个球体和一个点光源。我们将它们添加到了场景中。
4. 更新场景
接下来,您需要更新场景,以实现动态效果。您可以使用Vue.js的生命周期方法和requestAnimationFrame()函数来更新场景。
```javascript
mounted() {
// ...
// 开始渲染循环
this.renderLoop();
},
methods: {
renderLoop() {
// 更新场景元素
this.cube.rotation.x += 0.01;
this.cube.rotation.y += 0.01;
this.sphere.position.x = Math.sin(Date.now() * 0.001) * 2;
this.sphere.position.y = Math.cos(Date.now() * 0.001) * 2;
// 渲染场景
this.renderer.render(this.scene, this.camera);
// 请求下一帧
requestAnimationFrame(this.renderLoop);
}
}
```
在这个例子中,我们使用requestAnimationFrame()函数来更新场景。在renderLoop()方法中,我们更新了立方体和球体的位置和旋转。然后,我们使用渲染器渲染场景。
5. 添加交互
最后,您可以添加一些交互功能,例如用户点击或鼠标移动时的效果。您可以使用Vue.js的事件处理程序来处理这些事件。
```javascript
mounted() {
// ...
// 添加鼠标事件监听器
this.renderer.domElement.addEventListener('mousemove', this.onMouseMove);
},
methods: {
onMouseMove(event) {
// 计算鼠标位置
const mouse = new THREE.Vector2();
mouse.x = (event.clientX / window.innerWidth) * 2 - 1;
mouse.y = -(event.clientY / window.innerHeight) * 2 + 1;
// 更新相机位置
const cameraPosition = new THREE.Vector3();
cameraPosition.x = mouse.x * 10;
cameraPosition.y = mouse.y * 10;
cameraPosition.z = this.camera.position.z;
this.camera.position.copy(cameraPosition);
this.camera.lookAt(this.scene.position);
}
}
```
在这个例子中,我们添加了一个鼠标事件监听器。当鼠标移动时,我们计算鼠标位置,并使用它来更新相机位置。然后,我们使用lookAt()方法将相机对准场景中心。
这就是一个简单的Vue.js和Three.js的动态立体demo的实现方法。您可以使用这些基础知识来创建更复杂的可视化效果。
阅读全文
相关推荐


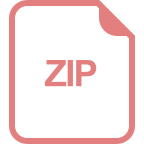
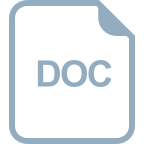

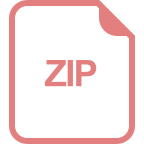
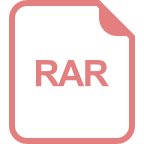
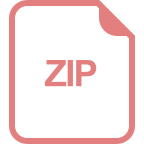
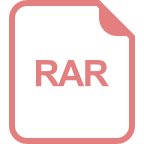
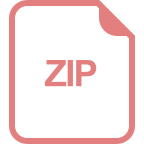
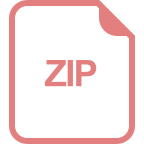
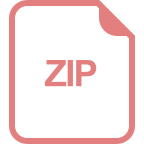
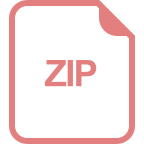
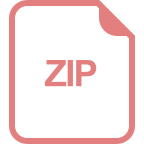
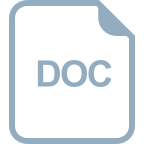
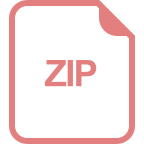
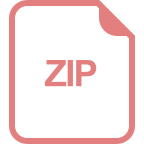