我输入两个链表以负数结束合并链表C语言
时间: 2024-03-13 09:16:16 浏览: 14
以下是一个示例程序,可以实现输入两个链表并合并它们:
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构体
struct ListNode {
int val;
struct ListNode *next;
};
// 创建新节点
struct ListNode* createNode(int val) {
struct ListNode* node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->val = val;
node->next = NULL;
return node;
}
// 合并两个链表
struct ListNode* mergeLists(struct ListNode* l1, struct ListNode* l2) {
struct ListNode dummy = {0, NULL};
struct ListNode* tail = &dummy;
while (l1 && l2) {
if (l1->val < l2->val) {
tail->next = l1;
l1 = l1->next;
} else {
tail->next = l2;
l2 = l2->next;
}
tail = tail->next;
}
tail->next = l1 ? l1 : l2;
return dummy.next;
}
// 释放链表内存
void freeList(struct ListNode* head) {
while (head) {
struct ListNode* node = head;
head = head->next;
free(node);
}
}
int main() {
struct ListNode *l1 = NULL, *l2 = NULL;
int val;
// 输入第一个链表
printf("Input the first list (separated by spaces, end with a negative number):\n");
while (scanf("%d", &val) == 1 && val >= 0) {
struct ListNode* node = createNode(val);
if (!l1) l1 = node;
else {
struct ListNode* tail = l1;
while (tail->next) tail = tail->next;
tail->next = node;
}
}
// 输入第二个链表
printf("Input the second list (separated by spaces, end with a negative number):\n");
while (scanf("%d", &val) == 1 && val >= 0) {
struct ListNode* node = createNode(val);
if (!l2) l2 = node;
else {
struct ListNode* tail = l2;
while (tail->next) tail = tail->next;
tail->next = node;
}
}
// 合并链表
struct ListNode* merged = mergeLists(l1, l2);
// 输出合并后的链表
printf("Merged list:\n");
struct ListNode* p = merged;
while (p) {
printf("%d ", p->val);
p = p->next;
}
printf("\n");
// 释放内存
freeList(l1);
freeList(l2);
freeList(merged);
return 0;
}
```
示例输入:
```
Input the first list (separated by spaces, end with a negative number):
1 3 5 7 9 -1
Input the second list (separated by spaces, end with a negative number):
2 4 6 8 10 -1
```
示例输出:
```
Merged list:
1 2 3 4 5 6 7 8 9 10
```
相关推荐
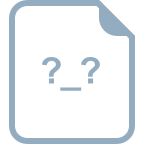
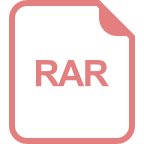














