一个链表将里面正数放在一个链表 负数放在另一个链表用c语言代码表示
时间: 2024-01-04 09:51:04 浏览: 85
以下是一个简单的例子,将正数放在一个链表,负数放在另一个链表:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
void insert(Node** head, int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = *head;
*head = newNode;
}
void splitList(Node* head, Node** positive, Node** negative) {
Node* current = head;
while (current != NULL) {
if (current->data >= 0) {
insert(positive, current->data);
} else {
insert(negative, current->data);
}
current = current->next;
}
}
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
Node* head = NULL;
insert(&head, 3);
insert(&head, -5);
insert(&head, 2);
insert(&head, -1);
insert(&head, 0);
insert(&head, 4);
Node* positive = NULL;
Node* negative = NULL;
splitList(head, &positive, &negative);
printf("Positive numbers: ");
printList(positive);
printf("Negative numbers: ");
printList(negative);
return 0;
}
```
在这个例子中,我们使用了三个函数:
- `insert()`:用于插入一个新节点到链表中。
- `splitList()`:用于将链表中的正数和负数分别插入到两个新的链表中。
- `printList()`:用于打印链表的所有元素。
我们首先创建了一个链表,然后调用 `splitList()` 函数将链表中的元素分别插入到两个新的链表中。最后,我们使用 `printList()` 函数打印两个新链表的所有元素。
阅读全文
相关推荐
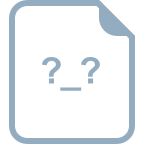
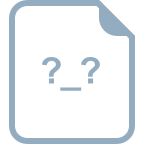


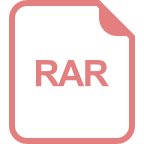
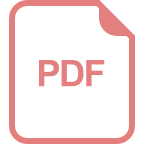
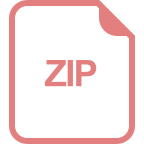
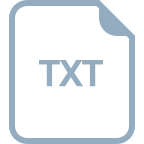
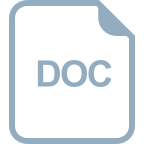
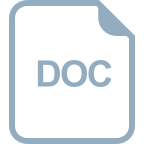
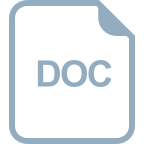
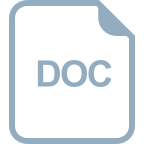





