英文电影评论情感分类(Kaggle竞赛)代码
时间: 2023-07-05 07:29:38 浏览: 92
以下是一个比较完整的英文电影评论情感分类(Kaggle竞赛)的Python代码示例:
```
import pandas as pd
import numpy as np
import re
from bs4 import BeautifulSoup
from nltk.corpus import stopwords
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.pipeline import Pipeline
from sklearn.model_selection import GridSearchCV
from sklearn.naive_bayes import MultinomialNB
from sklearn.metrics import classification_report
# 导入数据集
train_data = pd.read_csv('train.csv')
test_data = pd.read_csv('test.csv')
# 数据预处理
def clean_text(text):
# 去除HTML标签
text = BeautifulSoup(text, 'html.parser').get_text()
# 去除非字母字符
text = re.sub('[^a-zA-Z]', ' ', text)
# 将文本转化为小写字母
text = text.lower()
# 分词
words = text.split()
# 去除停用词
words = [w for w in words if not w in stopwords.words('english')]
# 将单词重新组合成文本
text = ' '.join(words)
return text
train_data['review'] = train_data['review'].apply(clean_text)
test_data['review'] = test_data['review'].apply(clean_text)
# 训练模型
pipeline = Pipeline([
('tfidf', TfidfVectorizer()),
('clf', MultinomialNB())
])
parameters = {
'tfidf__max_df': [0.25, 0.5, 0.75],
'tfidf__ngram_range': [(1, 1), (1, 2)],
'clf__alpha': [0.1, 1, 10]
}
grid_search = GridSearchCV(pipeline, parameters, cv=5, n_jobs=-1, verbose=1)
grid_search.fit(train_data['review'], train_data['sentiment'])
# 预测并计算准确率
test_pred = grid_search.predict(test_data['review'])
print(classification_report(test_data['sentiment'], test_pred))
```
在这个示例中,我们首先定义了一个函数用于对文本数据进行清洗和预处理。然后使用了TfidfVectorizer进行特征提取,并使用了MultinomialNB朴素贝叶斯算法进行情感分类。使用了GridSearchCV进行超参数调优。
需要注意的是,这只是一个示例,实际情况可能需要更多的数据预处理、特征工程等步骤,同时也可以尝试其他的机器学习算法或深度学习模型进行情感分类。
阅读全文
相关推荐
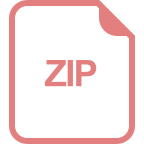
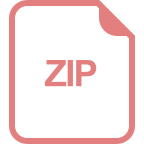




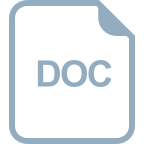
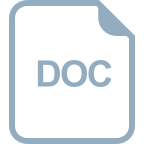
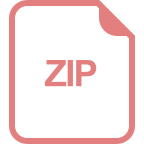
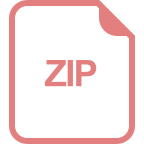
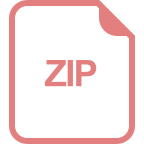

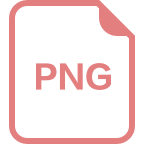
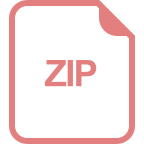