c 语言如何遍历多个文件夹0000-1000
时间: 2023-05-31 09:20:57 浏览: 107
### 回答1:
要遍历多个文件夹,可以使用递归函数来实现。具体步骤如下:
1. 定义一个函数,传入一个文件夹路径作为参数。
2. 在函数内部,使用 opendir() 函数打开文件夹,然后使用 readdir() 函数读取文件夹中的文件和子文件夹。
3. 对于每个读取到的文件或子文件夹,判断其类型,如果是文件夹,则递归调用该函数,传入该文件夹路径作为参数,以便继续遍历子文件夹。
4. 如果是文件,则进行相应的操作,比如读取文件内容等。
5. 遍历完当前文件夹后,使用 closedir() 函数关闭文件夹。
在主函数中,可以调用该函数多次,传入不同的文件夹路径,以遍历多个文件夹。
### 回答2:
在C语言中遍历多个文件夹的过程其实是一个遍历文件夹及其内部文件的过程,可以通过递归的方式进行实现。下面我将详细介绍如何使用C语言遍历多个文件夹(假设从0000到1000):
1. 定义遍历函数
首先,我们需要定义一个遍历函数,该函数可以接收文件夹路径作为参数,并遍历该文件夹中所有的文件和子文件夹。遍历函数可以使用递归的方式进行实现,以便能够遍历所有的文件夹和文件。函数的代码如下:
```c
#include <stdio.h>
#include <dirent.h>
#include <string.h>
void traverseFolder(const char *path){
DIR *dir;
struct dirent *entry;
char newPath[1024];
if((dir = opendir(path)) != NULL){
while((entry = readdir(dir)) != NULL){
if(entry->d_type == DT_DIR){
if(strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0){
continue;
}
snprintf(newPath, sizeof(newPath), "%s/%s", path, entry->d_name);
traverseFolder(newPath);
}else if(entry->d_type == DT_REG){
//如果是普通文件,可以根据需求自行处理
printf("File: %s/%s\n", path, entry->d_name);
}
}
closedir(dir);
}else{
printf("Open directory failed!\n");
}
}
```
2. 调用遍历函数遍历多个文件夹
接下来,我们需要根据需要遍历的文件夹数量,进行多次调用遍历函数,以遍历每个文件夹。如下所示:
```c
int main(){
char path[1024];
//从0000到1000遍历
for(int i = 0; i <= 1000; i++){
snprintf(path, sizeof(path), "./%04d", i);
traverseFolder(path);
}
return 0;
}
```
在主函数中,我们使用for循环进行了1001次循环,其中每次循环都将路径字符串进行格式化,并调用遍历函数进行遍历。由于路径是从0000到1000的,因此遍历了1001个文件夹。
3. 运行程序
最后,我们只需要在命令行中运行这个程序,就可以遍历多个文件夹了。需要注意的是,程序运行需要在包含要遍历的文件夹的目录下执行,否则会找不到文件夹。
以上就是使用C语言遍历多个文件夹的过程,通过以上步骤,我们可以轻松地遍历多个文件夹、获取每个文件夹中所有的文件和子文件夹。
### 回答3:
在 C 语言中遍历多个文件夹可以使用递归函数实现。首先需要包含头文件<dirent.h>,它定义了用于操作文件目录的函数。然后可以定义一个递归函数,在函数内部使用 opendir() 函数打开文件夹并使用 readdir() 函数读取文件夹中的内容。如果读到的是一个文件,则可以处理它;如果读到的是一个文件夹,则需要递归进入这个文件夹并继续遍历。
以下是一个示例代码:
```c
#include <stdio.h>
#include <dirent.h>
void traverse_dir(char* path)
{
DIR* dp = opendir(path);
struct dirent* entry;
while ((entry = readdir(dp)) != NULL) {
// 跳过 "." 和 ".." 目录
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) {
continue;
}
// 拼接文件路径
char full_path[2048];
snprintf(full_path, 2048, "%s/%s", path, entry->d_name);
if (entry->d_type == DT_DIR) { // 如果是文件夹,则递归进入
traverse_dir(full_path);
} else { // 如果是文件,则处理它
// 处理文件
printf("file: %s\n", full_path);
}
}
closedir(dp);
}
int main(int argc, char* argv[])
{
traverse_dir("0000-1000");
return 0;
}
```
在上面的代码中,traverse_dir() 函数接收一个文件夹路径作为参数,打开该文件夹并读取其中的内容,如果读到的是一个文件夹则递归调用 traverse_dir() 函数,如果读到的是一个文件则处理它。在 main() 函数中调用 traverse_dir() 函数,并传入初始的文件夹路径作为参数,即可遍历该文件夹下的所有文件。
相关推荐
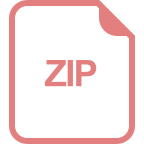
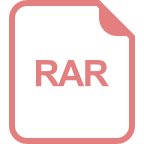
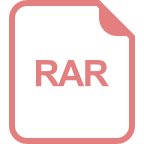














