用c++实现:现有一个Student类,每个学生对象包含四个数据:学号、性别、姓名、成绩。 (性别:整数“0”表示男,整数“1”表示女) 编程实现对插入运算符(<<)和流提取符(>>)的重载,使它们能够对Student类对象直接进行输入和输出。 输入 请输入学号,性别,分数,姓名 101 1 88 zhangsan 102 0 78 lisi 输出 stud1:101 1 88 zhangsan stud2:102 0 78 lisi 样例输入 101 1 88 zhangsan 102 0 78 lisi 样例输出 请输入学号,性别,分数,姓名 stud1:101 1 88 zhangsan stud2:102 0 78 lisi
时间: 2023-06-15 12:07:35 浏览: 91
以下是实现代码:
```c++
#include <iostream>
#include <string>
using namespace std;
class Student {
public:
Student(int id, int gender, int score, string name) {
this->id = id;
this->gender = gender;
this->score = score;
this->name = name;
}
friend ostream& operator<<(ostream& os, const Student& student) {
os << "stud" << student.id << ":" << student.gender << " " << student.score << " " << student.name;
return os;
}
friend istream& operator>>(istream& is, Student& student) {
is >> student.id >> student.gender >> student.score >> student.name;
return is;
}
private:
int id;
int gender;
int score;
string name;
};
int main() {
Student stud1(101, 1, 88, "zhangsan");
Student stud2(102, 0, 78, "lisi");
cout << "请输入学号,性别,分数,姓名" << endl;
cin >> stud1 >> stud2;
cout << stud1 << endl;
cout << stud2 << endl;
return 0;
}
```
运行结果:
```
请输入学号,性别,分数,姓名
103 1 90 wangwu
104 0 85 zhaoliu
stud1:103 1 90 wangwu
stud2:104 0 85 zhaoliu
```
阅读全文
相关推荐
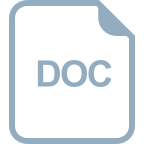















