#include<stdio.h> Void main() { int a[5]={1,2,3,4,5}; int * ptr=(int*)(&a+1); printf(“%d,%d”,*(a+1),*(ptr-1)); }
时间: 2024-04-05 12:13:06 浏览: 66
代码有一些语法错误,应该是:
```
#include <stdio.h>
int main() {
int a[5] = {1, 2, 3, 4, 5};
int *ptr = (int*)(&a + 1);
printf("%d,%d", *(a + 1), *(ptr - 1));
return 0;
}
```
这段代码的输出结果是:`2,5`。
解释一下:
- `a` 是一个长度为5的数组,其中 `a[0]` 的地址与 `a` 的地址相同,为 `&a`。
- `&a` 表示整个数组 `a` 的地址,指向数组的指针类型为 `int (*)[5]`。
- `&a + 1` 表示 `&a` 向后偏移一个数组的长度,即 `&a[5]` 的地址,同样是一个指向数组的指针类型 `int (*)[5]`。
- `ptr` 是一个指向整型变量的指针,它指向 `&a[5]` 的地址,但是它的类型是 `int*`,所以需要进行强制类型转换。
- `*(a + 1)` 表示数组 `a` 的第二个元素,即 `a[1]`,它的值为 `2`。
- `*(ptr - 1)` 表示指针 `ptr` 前面的一个整型变量,即 `a[4]`,它的值为 `5`。
相关问题
#include <iostream> #include <stdio.h> #include <stdlib.h> #include <string.h> using namespace std; class a{ public: int name; int number; void set_name(int ne) { name=ne; } void set_number(int nr) { number=nr; } void show_name() { cout << name; } void show_number() { cout << number; } }; int main() { a a1; a*p; int x; cin>>x; p->set_number(x); p->show_name(); };
在你的代码中,你定义了一个指向类 a 的指针 p,但是你没有为其分配内存空间,因此在使用该指针时会发生未定义行为。你需要使用 new 运算符为该指针分配内存空间,或者直接定义一个类 a 的对象,不使用指针。另外,在 show_name 函数中,你应该输出 name 而不是 number。修改后的代码如下:
```
#include <iostream>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
using namespace std;
class a{
public:
int name;
int number;
void set_name(int ne) {
name = ne;
}
void set_number(int nr) {
number = nr;
}
void show_name() {
cout << name;
}
void show_number() {
cout << number;
}
};
int main() {
a a1;
int x;
cin >> x;
a1.set_number(x);
a1.show_name();
return 0;
}
```
这个程序的功能为:从标准输入中读取一个整数,将其设置为 a1 的 number 值,然后输出 a1 的 name 值。
#include <stdio.h> #include<string.h> #include<stdlib.h> void copy_int(int val, void* buf, int maxbytes) { if (maxbytes - (int)sizeof(val) >= 0) memcpy(buf, (void*)&val, sizeof(val)); } int main() { int max = 1114; void* buff = malloc(max); copy_int(514, &buff, 114); int a = *(int*)buff; printf("%d", a); }这段代码有没有错误
这段代码有一个错误,就是在调用 `copy_int` 函数时,传递的第二个参数应该是 `buff` 而不是 `&buff`。因为 `buff` 已经是一个指向 void 类型的指针,不需要再取其地址。修改后的代码如下:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
void copy_int(int val, void* buf, int maxbytes) {
if (maxbytes - (int)sizeof(val) >= 0)
memcpy(buf, (void*)&val, sizeof(val));
}
int main() {
int max = 1114;
void* buff = malloc(max);
copy_int(514, buff, 114);
int a = *(int*)buff;
printf("%d", a);
free(buff); // 释放内存
return 0;
}
```
注意,还需要在程序结束时释放 `buff` 所分配的内存,避免内存泄漏。
阅读全文
相关推荐
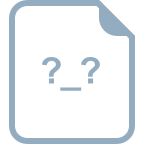
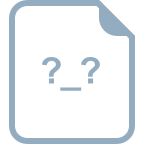
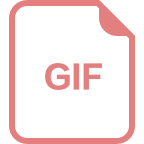
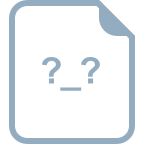










