图的邻接表创建、深度优先遍历和广度优先遍历代码实现
时间: 2023-06-21 17:09:26 浏览: 48
邻接表创建、深度优先遍历和广度优先遍历是图论中常见的操作,下面是 Python 代码实现:
邻接表创建:
```python
class Graph:
def __init__(self, vertices):
self.V = vertices
self.adj_list = [[] for _ in range(vertices)]
def add_edge(self, u, v):
self.adj_list[u].append(v)
self.adj_list[v].append(u)
```
深度优先遍历:
```python
def dfs_helper(graph, node, visited):
visited[node] = True
print(node, end=' ')
for neighbour in graph.adj_list[node]:
if not visited[neighbour]:
dfs_helper(graph, neighbour, visited)
def dfs(graph, start):
visited = [False] * graph.V
dfs_helper(graph, start, visited)
```
广度优先遍历:
```python
from queue import Queue
def bfs(graph, start):
visited = [False] * graph.V
q = Queue()
q.put(start)
visited[start] = True
while not q.empty():
node = q.get()
print(node, end=' ')
for neighbour in graph.adj_list[node]:
if not visited[neighbour]:
visited[neighbour] = True
q.put(neighbour)
```
其中,`graph` 是一个 `Graph` 类型的对象,`start` 是遍历的起始结点。
相关推荐
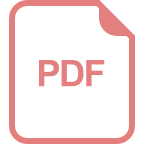
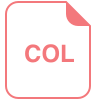
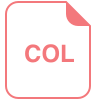
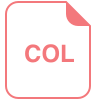
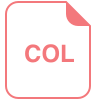
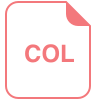









