定义一个StringUtils类,用String类的方法实现以下函数。 1、修改一个字符串中指定位置的字符。 2、在一个字符串的指定位置插入子串。 3、删除字符串中第一个指定的子串。 4、将字符串反序输出。
时间: 2023-05-11 16:03:50 浏览: 100
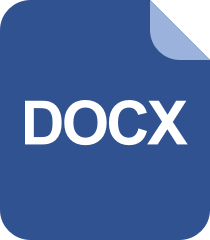
StringUtils的用法
1、修改一个字符串中指定位置的字符。
```
public class StringUtils {
public static String replaceChar(String str, int index, char newChar) {
if (str == null || index < 0 || index >= str.length()) {
return str;
}
char[] chars = str.toCharArray();
chars[index] = newChar;
return new String(chars);
}
}
```
2、在一个字符串的指定位置插入子串。
```
public class StringUtils {
public static String insertSubstring(String str, int index, String subStr) {
if (str == null || subStr == null || index < 0 || index > str.length()) {
return str;
}
StringBuilder sb = new StringBuilder(str);
sb.insert(index, subStr);
return sb.toString();
}
}
```
3、删除字符串中第一个指定的子串。
```
public class StringUtils {
public static String removeFirstSubstring(String str, String subStr) {
if (str == null || subStr == null) {
return str;
}
int index = str.indexOf(subStr);
if (index == -1) {
return str;
}
StringBuilder sb = new StringBuilder(str);
sb.delete(index, index + subStr.length());
return sb.toString();
}
}
```
4、将字符串反序输出。
```
public class StringUtils {
public static String reverse(String str) {
if (str == null) {
return str;
}
return new StringBuilder(str).reverse().toString();
}
}
```
阅读全文
相关推荐
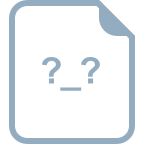
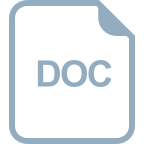



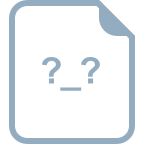
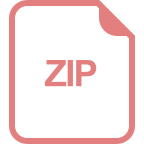
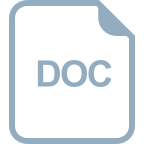
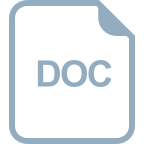
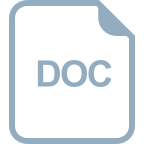
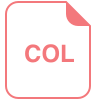
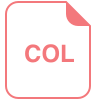
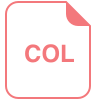
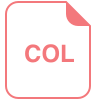
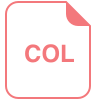
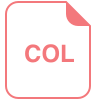
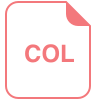
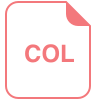