定义一个StringUtils类,用String类的方法实现以下函数。 1、修改一个字符串中指定位置的字符。
时间: 2023-05-11 12:03:47 浏览: 121
以下是定义一个StringUtils类,用String类的方法实现修改一个字符串中指定位置的字符的代码:
public class StringUtils {
public static String replaceChar(String str, int index, char newChar) {
if (str == null || index < 0 || index >= str.length()) {
return str;
}
char[] chars = str.toCharArray();
chars[index] = newChar;
return new String(chars);
}
}
这个类中的replaceChar方法可以接收一个字符串、一个索引和一个新字符作为参数,然后返回一个新的字符串,其中指定位置的字符被替换为新字符。如果传入的字符串为null或者索引越界,那么方法会直接返回原字符串。
相关问题
定义一个StringUtils类,用String类的方法实现以下函数。 1、修改一个字符串中指定位置的字符。 2、在一个字符串的指定位置插入子串。 3、删除字符串中第一个指定的子串。 4、将字符串反序输出。
1、修改一个字符串中指定位置的字符。
```
public class StringUtils {
public static String replaceChar(String str, int index, char newChar) {
if (str == null || index < 0 || index >= str.length()) {
return str;
}
char[] chars = str.toCharArray();
chars[index] = newChar;
return new String(chars);
}
}
```
2、在一个字符串的指定位置插入子串。
```
public class StringUtils {
public static String insertSubstring(String str, int index, String subStr) {
if (str == null || subStr == null || index < 0 || index > str.length()) {
return str;
}
StringBuilder sb = new StringBuilder(str);
sb.insert(index, subStr);
return sb.toString();
}
}
```
3、删除字符串中第一个指定的子串。
```
public class StringUtils {
public static String removeFirstSubstring(String str, String subStr) {
if (str == null || subStr == null) {
return str;
}
int index = str.indexOf(subStr);
if (index == -1) {
return str;
}
StringBuilder sb = new StringBuilder(str);
sb.delete(index, index + subStr.length());
return sb.toString();
}
}
```
4、将字符串反序输出。
```
public class StringUtils {
public static String reverse(String str) {
if (str == null) {
return str;
}
return new StringBuilder(str).reverse().toString();
}
}
```
定义一个stringutils类,用string方法实现以下函数
1. reverseString(string s):将字符串s反转并返回反转后的字符串。
2. isPalindrome(string s):判断字符串s是否为回文字符串(即正着读和反着读相同),返回true或false。
3. countOccurrences(string s, char c):计算字符c在字符串s中出现的次数并返回次数。
4. removeDuplicates(string s):去除字符串s中重复的字符并返回去重后的字符串。
5. toUpperCase(string s):将字符串s中所有字母转换为大写字母并返回转换后的字符串。
6. toLowerCase(string s):将字符串s中所有字母转换为小写字母并返回转换后的字符串。
7. capitalize(string s):将字符串s的首字母大写并返回修改后的字符串。
8. trim(string s):去除字符串s两端的空格,并返回去除空格后的字符串。
9. replaceAll(string s, string oldStr, string newStr):将字符串s中所有的oldStr替换为newStr,并返回替换后的字符串。
阅读全文
相关推荐
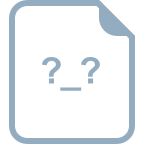

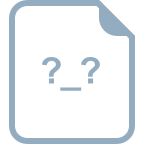
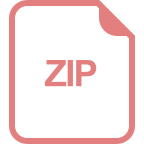
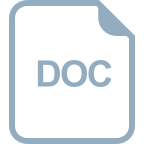
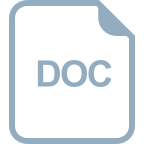
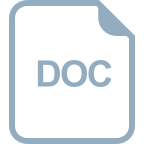
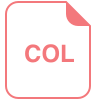
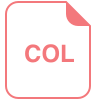
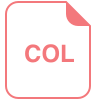
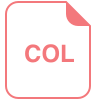
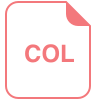
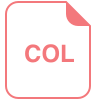
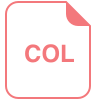
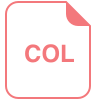