从键盘输入若干字符串,分别统计英文字母空格数字和其他字符的个数
时间: 2023-11-07 21:07:37 浏览: 39
好的,以下是实现代码:
```python
string = input("请输入字符串:")
letters = 0
spaces = 0
digits = 0
others = 0
for char in string:
if char.isalpha():
letters += 1
elif char.isspace():
spaces += 1
elif char.isdigit():
digits += 1
else:
others += 1
print("英文字母个数:", letters)
print("空格个数:", spaces)
print("数字个数:", digits)
print("其他字符个数:", others)
```
输入一个字符串后,程序会遍历字符串中的每个字符,通过 `isalpha()`、`isspace()`、`isdigit()` 判断字符类型并累加个数,最后输出统计结果。
相关问题
输入一行字符分别统计英文字母空格数字和其他字符的个数用C语言
以下是一个简单的 C 语言程序,可以输入一行字符并统计其中英文字母、空格、数字和其他字符的个数。
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int i, alpha, space, digit, other;
printf("请输入一行字符:\n");
fgets(str, sizeof(str), stdin);
alpha = space = digit = other = 0;
for (i = 0; str[i] != '\0'; i++) {
if (isalpha(str[i])) {
alpha++;
} else if (isspace(str[i])) {
space++;
} else if (isdigit(str[i])) {
digit++;
} else {
other++;
}
}
printf("英文字母个数:%d\n", alpha);
printf("空格个数:%d\n", space);
printf("数字个数:%d\n", digit);
printf("其他字符个数:%d\n", other);
return 0;
}
```
在程序中,我们定义了一个字符数组 `str`,用于存储输入的一行字符。然后,我们定义了四个变量 `alpha`、`space`、`digit` 和 `other`,分别表示英文字母、空格、数字和其他字符的个数,初始化为 0。
接下来,我们使用 `fgets()` 函数从标准输入中读取一行字符,并将其存储在 `str` 中。然后,我们使用一个循环来遍历这个字符串,对于每个字符,我们使用 `isalpha()`、`isspace()` 和 `isdigit()` 函数来判断它是英文字母、空格还是数字。如果不是这三种字符,则为其他字符。
最后,我们输出统计结果,即英文字母、空格、数字和其他字符的个数。
注意:上述程序中使用了 C 标准库中的 `isalpha()`、`isspace()` 和 `isdigit()` 函数,需要包含头文件 `<ctype.h>`。
python中输入一行字符串,分别统计其中英文字母 空格 数字和其它字符的个数
好的,下面是 Python 代码实现这个功能:
```python
s = input("请输入一行字符串:") # 获取用户输入的字符串
count_letter = 0 # 统计英文字母的个数
count_space = 0 # 统计空格的个数
count_digit = 0 # 统计数字的个数
count_other = 0 # 统计其他字符的个数
for c in s: # 遍历字符串中的每个字符
if c.isalpha(): # 如果是英文字母
count_letter += 1
elif c.isspace(): # 如果是空格
count_space += 1
elif c.isdigit(): # 如果是数字
count_digit += 1
else: # 否则是其他字符
count_other += 1
print("英文字母个数:", count_letter)
print("空格个数:", count_space)
print("数字个数:", count_digit)
print("其他字符个数:", count_other)
```
你可以直接复制这段代码到 Python 环境中运行。输入一行字符串,程序会统计其中英文字母、空格、数字和其他字符的个数,并输出结果。
相关推荐
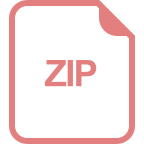
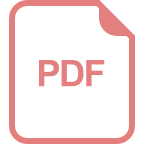
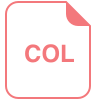
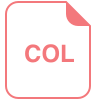
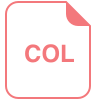









