Java中利用两个线程,其中一个实现将文件夹S中图片拷贝至文件夹D中,另一个将拷贝后图片名分别为a1,a2,a3.....的十张图片改名为1,2,3,。。
时间: 2023-12-06 15:44:26 浏览: 98
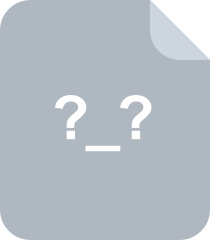
java实现对图片的各种操作

可以使用Java的多线程机制来实现这个功能。以下是一个简单的示例代码:
```java
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.StandardCopyOption;
public class ImageCopyAndRename {
public static void main(String[] args) throws IOException, InterruptedException {
// 源文件夹和目标文件夹路径
String srcDir = "S";
String destDir = "D";
// 创建拷贝线程和重命名线程
Thread copyThread = new Thread(() -> {
try {
copyImages(srcDir, destDir);
} catch (IOException e) {
e.printStackTrace();
}
});
Thread renameThread = new Thread(() -> {
try {
renameImages(destDir);
} catch (IOException e) {
e.printStackTrace();
}
});
// 启动线程
copyThread.start();
renameThread.start();
// 等待线程执行完毕
copyThread.join();
renameThread.join();
}
private static void copyImages(String srcDir, String destDir) throws IOException {
File[] files = new File(srcDir).listFiles();
if (files != null) {
for (File file : files) {
if (file.isFile()) {
Path destPath = new File(destDir, file.getName()).toPath();
Files.copy(file.toPath(), destPath, StandardCopyOption.REPLACE_EXISTING);
}
}
}
}
private static void renameImages(String destDir) throws IOException {
File[] files = new File(destDir).listFiles();
if (files != null) {
int i = 1;
for (File file : files) {
if (file.isFile()) {
Path oldPath = file.toPath();
Path newPath = new File(destDir, i + ".jpg").toPath();
Files.move(oldPath, newPath, StandardCopyOption.REPLACE_EXISTING);
i++;
}
}
}
}
}
```
在上面的代码中,我们创建了两个线程,一个用于将文件夹S中的图片拷贝到文件夹D中,另一个用于将拷贝后的图片重命名为1、2、3等等。在主线程中,我们启动这两个线程,并等待它们完成后退出程序。
其中,`copyImages`方法用于拷贝图片,`renameImages`方法用于重命名图片。这两个方法都使用了Java 7中引入的NIO.2 API,简化了文件操作。
需要注意的是,当拷贝图片时,需要保留原始文件名;当重命名图片时,需要将文件名改为数字。这里的示例代码假设所有图片都是jpg格式,如果有其他格式的图片,需要修改代码中的文件名后缀。
阅读全文
相关推荐
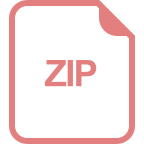

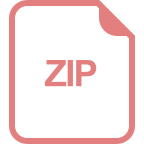
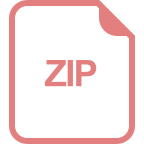
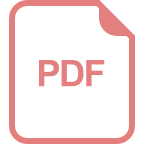
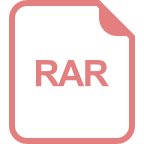
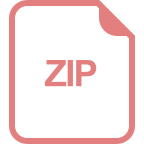
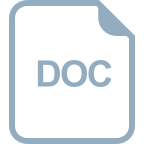
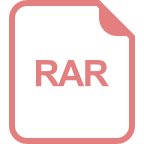
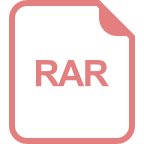
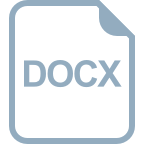
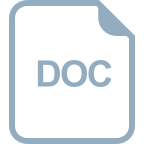
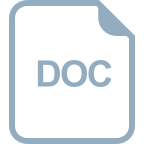




