python写跳棋的具体的移动和胜利检查逻辑
时间: 2024-03-05 19:47:37 浏览: 106
好的,下面我将为您介绍一些Python实现跳棋游戏中的具体移动和胜利检查逻辑。请注意,以下代码仅为示例,您可以根据自己的需求进行修改和扩展。
首先,让我们来看一下如何实现具体的移动逻辑。在跳棋游戏中,棋子可以在水平、垂直和对角线方向上移动,而且在跳跃过程中可以穿过其他棋子。因此,我们需要编写一个方法来检查给定的移动是否合法。下面是一个示例方法,可以检查从位置 `(x1, y1)` 移动到位置 `(x2, y2)` 是否合法:
```python
def is_valid_move(self, piece, end):
x1, y1 = piece.x, piece.y
x2, y2 = end
# check if the move is within the board
if x2 < 0 or x2 > 7 or y2 < 0 or y2 > 7:
return False
# check if the move is non-diagonal
if abs(x2 - x1) != abs(y2 - y1):
return False
# check if the move is longer than one square
if abs(x2 - x1) > 2:
return False
# check if the destination square is empty
if self.board.get_board()[x2][y2] != 0:
return False
# check if the move is a jump over an opponent's piece
if abs(x2 - x1) == 2:
mid_x, mid_y = (x1 + x2) // 2, (y1 + y2) // 2
if self.board.get_board()[mid_x][mid_y] == 0 or self.get_piece((mid_x, mid_y)).color == piece.color:
return False
return True
```
如果给定的移动是合法的,我们可以调用 `move` 方法来执行移动操作。`move` 方法会更新棋盘和棋子的状态,并且还会检查是否有棋子被吃掉。以下是一个示例方法:
```python
def move(self, start, end):
piece = self.get_piece(start)
if piece is not None and piece.color == self.turn:
if self.is_valid_move(piece, end):
piece.move(end)
self.board.move(start, end)
if abs(end[0] - start[0]) == 2:
mid_x, mid_y = (start[0] + end[0]) // 2, (start[1] + end[1]) // 2
self.pieces.remove(self.get_piece((mid_x, mid_y)))
return True
return False
```
接下来,让我们来看一下如何实现胜利检查逻辑。在跳棋游戏中,胜利条件是让对手无法再移动任何棋子。具体来说,如果对手所有棋子都被吃掉了或者所有棋子都无法移动,就算游戏结束。以下是一个示例方法,用于检查当前玩家是否获胜:
```python
def check_win(self):
for piece in self.pieces:
if piece.color == self.turn:
x, y = piece.x, piece.y
if self.is_valid_move(piece, (x+2, y+2)) or self.is_valid_move(piece, (x+2, y-2)) or \
self.is_valid_move(piece, (x-2, y+2)) or self.is_valid_move(piece, (x-2, y-2)):
return False
return True
```
在这个示例方法中,我们遍历当前玩家的所有棋子,检查是否有任何一个棋子可以移动到一个合法的位置。如果没有,就说明当前玩家无法移动任何棋子,游戏结束,当前玩家获胜。
希望这些代码能对您有所帮助!
阅读全文
相关推荐







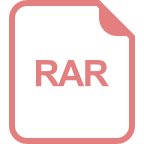










