跳棋小游戏python代码人机对战
时间: 2024-06-22 08:03:25 浏览: 14
编写一个简单的Python跳棋小游戏的人机对战,我们可以使用基本的控制台界面和简单的游戏逻辑。这里是一个基本框架的概述:
```python
# 导入必要的模块
import random
# 定义棋盘大小和初始棋子位置
BOARD_SIZE = 8
CHessPiece = ['X', 'O'] # X代表玩家,O代表电脑
def create_board():
board = [[' ' for _ in range(BOARD_SIZE)] for _ in range(BOARD_SIZE)]
return board
def place_piece(board, piece, position):
board[position][position] = piece
def check_win(board, piece):
# 检查四方向是否有连续的棋子
for dx, dy in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
for i in range(len(board) - 2):
if board[i][i + dx] == piece and board[i + 1][i + dx + dy] == piece and \
board[i + 2][i + dx + 2 * dy] == piece:
return True
return False
def make_move(board, player, AI_turn):
if AI_turn:
available_moves = find_available_moves(board, CHessPiece[1-player])
if available_moves:
return random.choice(available_moves)
else:
print("电脑无法移动,平局或你赢了!")
return None
else:
position = input(f"{player} 的回合,请输入你要落子的位置 (行, 列): ")
position = map(int, position.split(','))
return position
def main():
board = create_board()
player = 'X'
AI_turn = True
while True:
place_piece(board, CHessPiece[player], make_move(board, player, AI_turn))
if check_win(board, CHessPiece[player]):
print(f"{player} 赢了!")
break
if not AI_turn:
AI_turn = True
else:
AI_turn = False
player = 'X' if player == 'O' else 'O'
if __name__ == "__main__":
main()
```
相关推荐
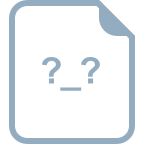














