帮我写一个由python subprocess库远程操控虚拟机centos 执行onlineSwitch.sh脚本
时间: 2024-02-01 15:02:42 浏览: 37
好的,以下是一个使用 Python subprocess 模块远程操控 CentOS 虚拟机并执行 onlineSwitch.sh 脚本的示例代码:
```python
import subprocess
# CentOS 虚拟机的 IP 地址
ip_address = "192.168.1.100"
# 在 CentOS 虚拟机上执行 onlineSwitch.sh 脚本的命令
command = "ssh root@{} 'sh /path/to/onlineSwitch.sh'".format(ip_address)
# 执行命令并获取输出结果
result = subprocess.run(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
# 输出命令执行结果
print(result.stdout.decode("utf-8"))
```
以上代码中,我们首先定义了 CentOS 虚拟机的 IP 地址,并构建了一个执行 onlineSwitch.sh 脚本的命令,然后使用 Python subprocess 模块的 `run` 方法执行该命令,并获取输出结果,最后将输出结果打印出来。请注意将代码中的 `ip_address` 和 `/path/to/onlineSwitch.sh` 替换为实际的 IP 地址和脚本路径。另外,需要确保在 CentOS 虚拟机上已经安装了 SSH 服务。
相关问题
帮我写一个远程虚拟机执行sh /text/onlineSwitch.sh
好的,你可以使用下面的 Python 代码来执行远程虚拟机上的 `sh /text/onlineSwitch.sh` 命令:
```python
import subprocess
# 远程虚拟机的 IP 地址
remote_host = '192.168.0.100'
# 远程执行的命令
remote_cmd = 'sh /text/onlineSwitch.sh'
# 使用 SSH 连接远程主机,并执行命令
ssh_cmd = f'ssh {remote_host} "{remote_cmd}"'
result = subprocess.run(ssh_cmd, shell=True, check=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
# 输出执行结果
print(result.stdout.decode())
```
在这个代码中,我们使用 `subprocess.run()` 函数来执行远程 SSH 命令,其中 `remote_host` 变量是远程虚拟机的 IP 地址,`remote_cmd` 变量是要执行的命令。然后,我们将这两个变量拼接成一个完整的 SSH 命令,并使用 `subprocess.run()` 函数来执行它。最后,我们使用 `print()` 函数将执行结果打印出来。
如何使用ssh_comand 封装 onlineSwitch.sh
假设您想要在远程服务器上执行一个名为`onlineSwitch.sh`的脚本,您可以使用`ssh_command`封装该命令,并使用`os.system`或`subprocess`模块来执行。以下是一个示例代码:
```python
import os
# 设置SSH连接信息
host = 'remote.host.com'
user = 'remoteuser'
password = 'remotepass'
# 建立SSH连接
ssh_command = f'sshpass -p {password} ssh {user}@{host}'
# 在远程服务器上执行命令
command = './onlineSwitch.sh'
os.system(f'{ssh_command} "{command}"')
```
在这个示例中,我们使用`ssh_command`封装了SSH连接命令,然后使用`os.system`执行`./onlineSwitch.sh`命令。需要注意的是,如果`onlineSwitch.sh`脚本不在当前目录下,您需要使用`cd`命令进入该脚本所在的目录,然后再执行该脚本。
如果您需要在Python程序中获取`onlineSwitch.sh`脚本的输出,可以使用`subprocess`模块中的`check_output`函数。以下是一个示例代码:
```python
import subprocess
# 设置SSH连接信息
host = 'remote.host.com'
user = 'remoteuser'
password = 'remotepass'
# 在远程服务器上执行命令,获取输出
command = './onlineSwitch.sh'
output = subprocess.check_output(['sshpass', '-p', password, 'ssh', f'{user}@{host}', command])
# 打印输出
print(output.decode('utf-8'))
```
在这个示例中,我们使用`subprocess.check_output`函数执行`./onlineSwitch.sh`命令,并将输出保存在`output`变量中。需要注意的是,`subprocess.check_output`函数的第一个参数是一个列表,其中包含了需要执行的命令及其参数。然后,我们将输出解码为UTF-8格式,并打印输出。
阅读全文
相关推荐
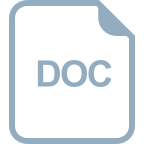
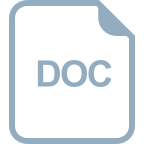
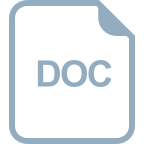
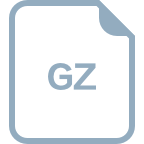
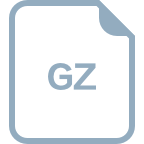







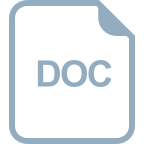
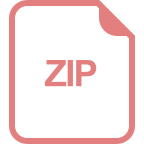