python实现学生通讯录管理系统
时间: 2023-09-09 17:11:58 浏览: 278
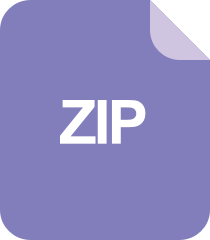
课程设计--Python通讯录管理系统.zip

好的,下面是一个简单的学生通讯录管理系统的Python实现。
```python
# 定义学生类
class Student:
def __init__(self, name, age, phone):
self.name = name
self.age = age
self.phone = phone
# 定义通讯录管理类
class ContactManage:
def __init__(self):
self.students = []
# 添加学生
def add_student(self, student):
self.students.append(student)
# 删除学生
def delete_student(self, name):
for student in self.students:
if student.name == name:
self.students.remove(student)
# 修改学生信息
def modify_student(self, name, age, phone):
for student in self.students:
if student.name == name:
student.age = age
student.phone = phone
# 查询学生信息
def query_student(self, name):
for student in self.students:
if student.name == name:
print("姓名:{},年龄:{},电话:{}".format(student.name, student.age, student.phone))
# 显示所有学生信息
def show_all_students(self):
for student in self.students:
print("姓名:{},年龄:{},电话:{}".format(student.name, student.age, student.phone))
# 测试
if __name__ == '__main__':
contact_manage = ContactManage()
student1 = Student('张三', 18, '123456789')
student2 = Student('李四', 20, '987654321')
contact_manage.add_student(student1)
contact_manage.add_student(student2)
contact_manage.show_all_students()
contact_manage.modify_student('张三', 19, '111111111')
contact_manage.show_all_students()
contact_manage.delete_student('李四')
contact_manage.show_all_students()
contact_manage.query_student('张三')
```
这个程序通过定义学生类和通讯录管理类来实现学生通讯录管理系统。可以添加、删除、修改、查询学生信息,以及显示所有学生信息。当然,这只是一个简单的示例,实际使用时还需要根据具体需求进行修改和扩展。
阅读全文
相关推荐
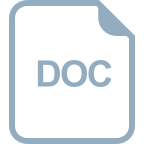
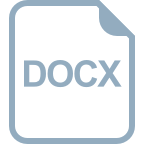
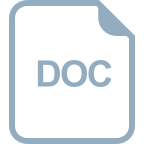
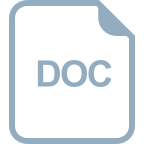
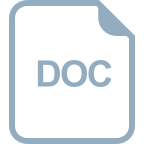









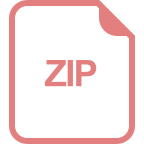
