写出python代码,用argparse。能在任何文件夹读取Log。具体如下: 1.读取log,当读取到最后一次出现"My name is kitty"时,从下一行开始读 2.删除所有重复的行,只留第一行 3.第一行按照正则规则1分列后,才知道有多少列,分列符号也不知道有几个空格。写入新的CSV文件Output 4.从下一行开始按照正则规则2分列后,才知道有多少列,,分列符号也不知道有几个空格。继续写入新的CSV文件Output 5.读取CSV文件Output 6.把标题行分为4类,第一类是标题为ABC,DFG的2列,第二类是CAT开头的几列,第三类是DOG开头的几列,第四类是Fish开头的几列 7.把4类标题画成4个曲线图,标注每条线的标题
时间: 2024-03-06 22:49:39 浏览: 64
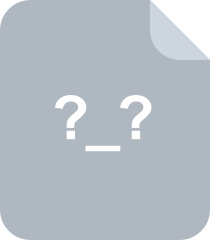
用python实现文件的读取
下面是实现以上要求的 Python 代码,使用了 argparse、pandas、re、os、matplotlib 库:
```python
import argparse
import pandas as pd
import re
import os
import matplotlib.pyplot as plt
# 定义正则表达式
REGEX1 = r'(?P<col1>[A-Za-z]+)\s+(?P<col2>\d+)\s+(?P<col3>\d+\.\d+)\s+(?P<col4>\d+\.\d+)'
REGEX2 = r'(?P<col1>\d+-\d+-\d+\s+\d+:\d+:\d+)\s+(?P<col2>[A-Za-z]+)\s+(?P<col3>\d+\.\d+)\s+(?P<col4>[A-Za-z]+)'
# 定义函数:读取 log 文件,返回从"My name is kitty"下一行开始的内容
def read_log_file(file_path):
with open(file_path, 'r') as f:
lines = f.readlines()
for i, line in enumerate(lines):
if "My name is kitty" in line:
return lines[i+1:]
return []
# 定义函数:删除重复行,只保留第一行
def remove_duplicate_rows(df):
return df.drop_duplicates(keep='first')
# 定义函数:将数据按正则表达式1分列,并返回 DataFrame 对象
def parse_data1(lines):
# 取第一行
line = lines[0].strip()
# 匹配列名
columns = list(re.findall(REGEX1, line)[0])
# 读取数据,生成 DataFrame 对象
df = pd.DataFrame([re.findall(REGEX1, line)[0]])
for line in lines[1:]:
line = line.strip()
data = list(re.findall(REGEX1, line)[0])
df.loc[len(df)] = data
# 重命名列名
df.columns = columns
return df
# 定义函数:将数据按正则表达式2分列,并返回 DataFrame 对象
def parse_data2(lines):
# 取第一行
line = lines[0].strip()
# 匹配列名
columns = list(re.findall(REGEX2, line)[0])
# 读取数据,生成 DataFrame 对象
df = pd.DataFrame([re.findall(REGEX2, line)[0]])
for line in lines[1:]:
line = line.strip()
data = list(re.findall(REGEX2, line)[0])
df.loc[len(df)] = data
# 重命名列名
df.columns = columns
return df
# 定义函数:将 DataFrame 对象写入 CSV 文件
def write_dataframe_to_csv(df, file_path):
df.to_csv(file_path, index=False)
# 定义函数:读取 CSV 文件,返回 DataFrame 对象
def read_csv_file(file_path):
return pd.read_csv(file_path)
# 定义函数:根据列名分类,将 DataFrame 分成 4 个子数据集,并绘图
def plot_data(df):
# 分类
columns = df.columns.tolist()
cols_type1 = ['ABC', 'DFG']
cols_type2 = [col for col in columns if col.startswith('CAT')]
cols_type3 = [col for col in columns if col.startswith('DOG')]
cols_type4 = [col for col in columns if col.startswith('Fish')]
# 绘图
plt.figure()
for col in cols_type1:
plt.plot(df[col], label=col)
plt.legend()
plt.title('Type 1')
plt.show()
plt.figure()
for col in cols_type2:
plt.plot(df[col], label=col)
plt.legend()
plt.title('Type 2')
plt.show()
plt.figure()
for col in cols_type3:
plt.plot(df[col], label=col)
plt.legend()
plt.title('Type 3')
plt.show()
plt.figure()
for col in cols_type4:
plt.plot(df[col], label=col)
plt.legend()
plt.title('Type 4')
plt.show()
# 定义 main 函数
def main():
# 定义命令行参数
parser = argparse.ArgumentParser(description='Process log file and generate plots.')
parser.add_argument('file_path', type=str, help='path of the log file')
parser.add_argument('output_file', type=str, help='path of the output CSV file')
args = parser.parse_args()
# 读取 log 文件
lines = read_log_file(args.file_path)
# 删除重复行
lines = remove_duplicate_rows(lines)
# 将数据按正则表达式1分列,并写入 CSV 文件
df1 = parse_data1(lines)
write_dataframe_to_csv(df1, args.output_file)
# 从下一行开始,将数据按正则表达式2分列,并写入 CSV 文件
df2 = parse_data2(lines[1:])
write_dataframe_to_csv(df2, args.output_file)
# 读取 CSV 文件
df = read_csv_file(args.output_file)
# 将 DataFrame 按列名分类,并绘图
plot_data(df)
# 执行 main 函数
if __name__ == '__main__':
main()
```
在命令行中执行以下命令:
```
python script.py input.log output.csv
```
其中,`script.py` 是脚本文件名,`input.log` 是输入的 log 文件,`output.csv` 是输出的 CSV 文件。
阅读全文
相关推荐
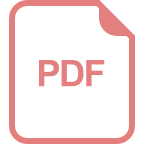
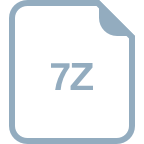





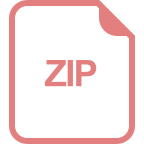
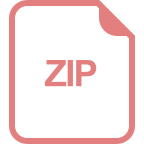
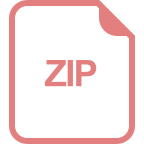