给我一段Hash256的Java源代码
时间: 2023-09-20 17:06:03 浏览: 130
### 回答1:
下面是一段使用 Java 实现的 Hash256 的源代码:
```
import java.security.MessageDigest;
public class Hash256 {
public static String hash256(String data) throws Exception {
MessageDigest md = MessageDigest.getInstance("SHA-256");
md.update(data.getBytes());
return bytesToHex(md.digest());
}
public static String bytesToHex(byte[] bytes) {
StringBuffer result = new StringBuffer();
for (byte b : bytes) result.append(Integer.toString((b & 0xff) + 0x100, 16).substring(1));
return result.toString();
}
}
```
使用这个类的方法是调用 `hash256(String data)`,其中 `data` 是要被哈希的字符串。这个方法会返回一个十六进制的哈希字符串。
例如,要对字符串 "Hello, world!" 进行哈希,你可以这样调用这个方法:
```
String hash = Hash256.hash256("Hello, world!");
```
这样,`hash` 变量就包含了对字符串 "Hello, world!" 进行 Hash256 哈希后的结果。
### 回答2:
以下是一个简单的Hash256的Java源代码示例:
```java
import java.nio.charset.StandardCharsets;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class Hash256Example {
public static void main(String[] args) throws NoSuchAlgorithmException {
String input = "Hello, world!"; // 要进行Hash的输入字符串
String hashResult = getHash256(input);
System.out.println("Hash结果: " + hashResult);
}
public static String getHash256(String input) throws NoSuchAlgorithmException {
MessageDigest digest = MessageDigest.getInstance("SHA-256");
byte[] hash = digest.digest(input.getBytes(StandardCharsets.UTF_8));
StringBuilder hexString = new StringBuilder();
for (byte b : hash) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) {
hexString.append('0');
}
hexString.append(hex);
}
return hexString.toString();
}
}
```
这个代码示例使用了Java的`MessageDigest`类和SHA-256算法来进行Hash计算。`getHash256`方法接受一个字符串作为输入,通过调用`MessageDigest`类的`digest`方法来计算出输入的SHA-256哈希值。然后,将哈希值转换为十六进制字符串表示并返回。在`main`方法中,我们给定了一个输入字符串并调用`getHash256`方法来获取它的SHA-256哈希结果。最后,将结果打印输出到控制台。
### 回答3:
下面是一个例子展示如何使用Java编写一个Hash256的源代码:
```java
import java.nio.charset.StandardCharsets;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class Hash256Example {
public static void main(String[] args) {
String input = "Hello World";
try {
byte[] hash = getHash256(input);
// 将字节数组转换为十六进制字符串
StringBuilder hexString = new StringBuilder();
for (byte b : hash) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) {
hexString.append('0');
}
hexString.append(hex);
}
System.out.println("Hash256值为:" + hexString.toString());
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
}
public static byte[] getHash256(String input) throws NoSuchAlgorithmException {
MessageDigest digest = MessageDigest.getInstance("SHA-256");
return digest.digest(input.getBytes(StandardCharsets.UTF_8));
}
}
```
以上代码展示了如何使用Java中的MessageDigest类来实现SHA-256哈希算法。在示例中,我们传入字符串"Hello World"作为输入,并使用getHash256方法计算它的Hash256值。最后,我们将字节数组转换为十六进制字符串并打印出来。请注意,还需要处理NoSuchAlgorithmException,在catch块中输出异常堆栈跟踪信息。
阅读全文
相关推荐


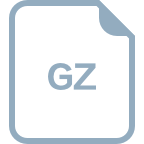




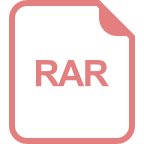
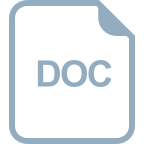









