c++中实现websocket生产端和服务端
时间: 2024-01-21 08:16:12 浏览: 34
在C++中,可以使用第三方库来实现WebSocket生产端和服务端。常用的WebSocket库有:libwebsockets、uWebSockets和Boost.Beast等。
以下是使用libwebsockets库实现WebSocket生产端和服务端的示例代码:
WebSocket服务端:
```c++
#include <libwebsockets.h>
#include <string.h>
static int callback_echo(struct libwebsocket_context *context,
struct libwebsocket *wsi,
enum libwebsocket_callback_reasons reason,
void *user, void *in, size_t len)
{
switch (reason) {
case LWS_CALLBACK_ESTABLISHED:
printf("Connection established\n");
break;
case LWS_CALLBACK_RECEIVE:
// 从客户端接收到消息
printf("Received message: %s\n", (char *) in);
// 回复消息给客户端
libwebsocket_write(wsi, (unsigned char *) in, strlen((char *) in), LWS_WRITE_TEXT);
break;
default:
break;
}
return 0;
}
int main(int argc, char **argv)
{
struct libwebsocket_context *context;
struct libwebsocket_protocols protocols[] = {
{ "echo-protocol", callback_echo, 0 },
{ NULL, NULL, 0 }
};
struct lws_context_creation_info info;
memset(&info, 0, sizeof info);
info.port = 9000;
info.protocols = protocols;
context = libwebsocket_create_context(&info);
if (context == NULL) {
printf("Could not create WebSocket context\n");
return 1;
}
printf("WebSocket server started\n");
while (1) {
libwebsocket_service(context, 50);
}
libwebsocket_context_destroy(context);
return 0;
}
```
WebSocket生产端:
```c++
#include <libwebsockets.h>
#include <string.h>
static void connect_callback(struct libwebsocket_context *context,
struct libwebsocket *wsi,
enum libwebsocket_callback_reasons reason,
void *user, void *in, size_t len)
{
if (reason == LWS_CALLBACK_CLIENT_CONNECTION_ERROR) {
printf("Connection failed\n");
exit(1);
}
if (reason == LWS_CALLBACK_CLIENT_ESTABLISHED) {
printf("Connection established\n");
// 发送消息给服务端
char *message = "Hello, WebSocket server!";
libwebsocket_write(wsi, (unsigned char *) message, strlen(message), LWS_WRITE_TEXT);
}
}
int main(int argc, char **argv)
{
struct lws_context_creation_info info;
memset(&info, 0, sizeof info);
info.port = CONTEXT_PORT_NO_LISTEN;
info.protocols = NULL;
struct libwebsocket_context *context = libwebsocket_create_context(&info);
if (context == NULL) {
printf("Could not create WebSocket context\n");
return 1;
}
struct libwebsocket *wsi = libwebsocket_client_connect(context, "localhost", 9000,
0, "/", "localhost", NULL, NULL, -1);
if (wsi == NULL) {
printf("Could not connect to WebSocket server\n");
return 1;
}
printf("Connecting to WebSocket server...\n");
while (1) {
libwebsocket_service(context, 50);
}
libwebsocket_context_destroy(context);
return 0;
}
```
注意:以上示例代码仅供参考,实际使用时需要根据具体需求进行修改。
相关推荐
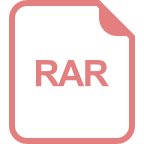
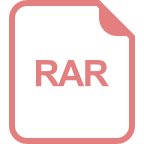
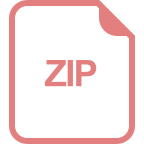














