任务描述 用C++编程 设计一个简单的学生类(Student) 学生类(Student): 公有数据成员:姓名(name),字符数组 公有数据成员:编号(id),整数类型,请借助totalCount自动计算学生的编号 私有数据成员:年龄(age),整数类型 静态数据成员:总人数(totalCount),整数类型,用于记录学生对象的总数 构造函数:只允许接受姓名和年龄作为参数,将其赋值给对应的成员变量,并将总人数加一 公有成员函数:displayInfo(),用于显示学生的姓名、年龄、编号和总人数,格式:name: Liam, age: 23, id: 1, totalCount: 1 要求: 输入格式:第一行输入一个整数k说明将有k个学生,第2到k+1行输入格式为:name age 输出格式:name: Liam, age: 23, id: 1, totalCount: 1 请注意每轮输入后需要将totalCount归零 题目确保姓名不会超过20个字,可以直接将name字符数组的长度设置为20,char* name = new char[20]; 样例输入: 5 Liam 23 Olivia 26 Noah 23 Emma 11 Oliver 12 样例输出: name: Liam, age: 23, id: 1, totalCount: 1 name: Olivia, age: 26, id: 2, totalCount: 2 name: Noah, age: 23, id: 3, totalCount: 3 name: Emma, age: 11, id: 4, totalCount: 4 name: Oliver, age: 12, id: 5, totalCount: 5 样例输入: 3 Ava 24 Elijah 12 Isabella 19 样例输出: name: Ava, age: 24, id: 1, totalCount: 1 name: Elijah, age: 12, id: 2, totalCount: 2 name: Isabella, age: 19, id: 3, totalCount: 3 样例输入: 4 Lucas 22 Sophia 24 Mason 12 Mia 29 样例输出: name: Lucas, age: 22, id: 1, totalCount: 1 name: Sophia, age: 24, id: 2, totalCount: 2 name: Mason, age: 12, id: 3, totalCount: 3 name: Mia, age: 29, id: 4, totalCount: 4
时间: 2024-02-03 21:03:59 浏览: 76
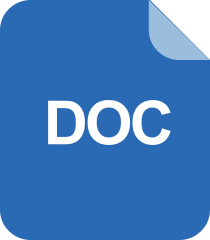
设计一个学生类(CStudent)

以下是本题的C++代码实现:
```cpp
#include<iostream>
using namespace std;
class Student{
public:
char* name = new char[20];
int id;
int age;
static int totalCount;
Student(char* n, int a){
id = ++totalCount;
age = a;
for(int i = 0; i < 20; i++){
name[i] = n[i];
}
totalCount++;
}
void displayInfo(){
cout << "name: " << name << ", age: " << age << ", id: " << id << ", totalCount: " << totalCount << endl;
}
};
int Student::totalCount = 0;
int main(){
int k;
cin >> k;
for(int i = 0; i < k; i++){
char* n = new char[20];
int a;
cin >> n >> a;
Student s(n, a);
s.displayInfo();
Student::totalCount = 0;
}
return 0;
}
```
解析:
1. 首先定义 `Student` 类,其中包含公有数据成员 `name` 和 `id`,私有数据成员 `age`,静态数据成员 `totalCount`,以及构造函数 `Student` 和成员函数 `displayInfo`。
2. 构造函数 `Student` 接收两个参数 `n` 和 `a`,将其分别赋值给成员变量 `name` 和 `age`,并自动计算出学生的编号 `id`,并将总人数 `totalCount` 加一。
3. 成员函数 `displayInfo` 用于显示学生的姓名、年龄、编号和总人数,格式为 `name: Liam, age: 23, id: 1, totalCount: 1`。
4. 在 `main` 函数中,首先读入学生数量 `k`,然后循环读入每个学生的姓名和年龄,并创建一个 `Student` 对象,调用其成员函数 `displayInfo` 显示信息。注意,在每次循环结束后需要将总人数 `totalCount` 归零。
阅读全文
相关推荐
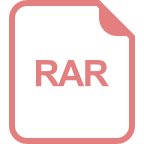
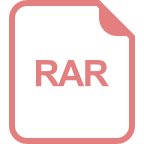
















