C++ 1.设计一个简单的学生类 请设计一个名为 Student 的类,包含学生的姓名(name)、学号(id)和年龄(age)作私有成员变量。为这些私有成员变量提供公有成员函数以设置 (setter)和获取(getter)它们的值。此外
时间: 2024-09-20 21:16:03 浏览: 36
在C++中,我们可以按照下面的方式来设计一个简单的`Student`类:
```cpp
#include <string>
class Student {
private:
std::string name; // 学生姓名
int id; // 学号
int age; // 年龄
public:
// 构造函数
Student(const std::string& studentName, int studentId, int studentAge)
: name(studentName), id(studentId), age(studentAge) {}
// 公有成员函数 - 设置姓名
void setName(const std::string& newName) {
name = newName;
}
// 公有成员函数 - 获取姓名
const std::string& getName() const {
return name;
}
// 公有成员函数 - 设置学号
void setID(int newId) {
id = newId;
}
// 公有成员函数 - 获取学号
int getID() const {
return id;
}
// 公有成员函数 - 设置年龄
void setAge(int newAge) {
if (newAge >= 0) { // 防止设置负数年龄
age = newAge;
}
}
// 公有成员函数 - 获取年龄
int getAge() const {
return age;
}
};
```
在这个例子中,我们创建了一个`Student`类,包含了三个私有成员变量`name`、`id`和`age`,并分别为每个变量提供了对应的 getter 和 setter 函数。这样,外部代码就可以通过对象的公共接口安全地访问和修改学生的信息了。
相关推荐
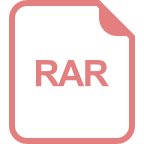
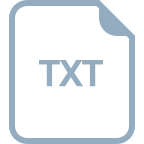
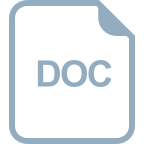














