定义一个学生类student,类有五个数据成员(学号、姓名、年龄、C++及高数两科成绩),以及一下成员函数。请编写主函数使用这个类,实现对学生数据的赋值和输出,要求如下:1.设计两个成员函数分别实现对数据的输入、输出。2.设计一个构造函数实现初始化或传递相关数据。3.设计一个析构函数,能显示相应信息。4.设计一个成员函数计算总成绩并输出。表明你的设计思想及实现步骤
时间: 2024-02-26 18:52:29 浏览: 81
好的,我可以帮你解决这个问题。首先,我们需要定义一个学生类student,可以在类中定义以下数据成员和成员函数:
```cpp
class student {
public:
student(int id, string name, int age, double cpp_score, double math_score); // 构造函数
~student(); // 析构函数
void input(); // 输入数据的函数
void output(); // 输出数据的函数
void calculate_total_score(); // 计算总成绩的函数
private:
int id; // 学号
string name; // 姓名
int age; // 年龄
double cpp_score; // C++成绩
double math_score; // 高数成绩
double total_score; // 总成绩
};
```
在类中,我们定义了五个数据成员:学号、姓名、年龄、C++及高数两科成绩,以及一个计算总成绩的数据成员。同时,我们定义了以下成员函数:
- 构造函数:用于初始化或传递相关数据。
- 析构函数:能够在对象被销毁时,显示相应信息。
- 输入数据的函数:用于输入学生的信息。
- 输出数据的函数:用于输出学生的信息。
- 计算总成绩的函数:用于计算学生的总成绩。
下面是具体的实现代码:
```cpp
#include <iostream>
#include <string>
using namespace std;
class student {
public:
student(int id, string name, int age, double cpp_score, double math_score) {
this->id = id;
this->name = name;
this->age = age;
this->cpp_score = cpp_score;
this->math_score = math_score;
}
~student() {
cout << "学生 " << name << " 的信息已经销毁" << endl;
}
void input() {
cout << "请输入学生的学号:" << endl;
cin >> id;
cout << "请输入学生的姓名:" << endl;
cin >> name;
cout << "请输入学生的年龄:" << endl;
cin >> age;
cout << "请输入学生的C++成绩:" << endl;
cin >> cpp_score;
cout << "请输入学生的高数成绩:" << endl;
cin >> math_score;
}
void output() {
cout << "学生的学号是:" << id << endl;
cout << "学生的姓名是:" << name << endl;
cout << "学生的年龄是:" << age << endl;
cout << "学生的C++成绩是:" << cpp_score << endl;
cout << "学生的高数成绩是:" << math_score << endl;
cout << "学生的总成绩是:" << total_score << endl;
}
void calculate_total_score() {
total_score = cpp_score + math_score;
}
private:
int id; // 学号
string name; // 姓名
int age; // 年龄
double cpp_score; // C++成绩
double math_score; // 高数成绩
double total_score; // 总成绩
};
int main() {
student s(0, "", 0, 0, 0);
s.input();
s.calculate_total_score();
s.output();
return 0;
}
```
在主函数中,我们首先定义一个学生对象s,然后通过调用s的input函数输入学生的信息,并通过调用s的calculate_total_score函数计算学生的总成绩,最后通过调用s的output函数输出学生的信息,包括总成绩。
这样,我们就实现了一个简单的学生类,通过类的数据成员和成员函数,实现了对学生信息的输入、计算和输出。
阅读全文
相关推荐



















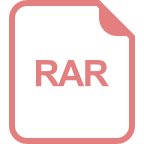